Table of Contents
ToggleDot NET interview questions For Freshers
Here are 40 important .NET interview questions along with their answers for freshers. These cover basic to intermediate .NET concepts, C#, ASP.NET, ADO.NET, and other essential topics.
Basic .NET Framework & Concepts
What is the .NET Framework?
- Answer: The .NET Framework is a software development platform developed by Microsoft. It includes a large class library (Base Class Library) and provides runtime support via the Common Language Runtime (CLR). It is primarily used for building Windows-based applications and web applications.
What is the difference between .NET Framework and .NET Core?
- Answer:
- .NET Framework: A Windows-only platform used for building Windows applications and web services.
- .NET Core: A cross-platform, open-source framework that supports multiple operating systems (Windows, Linux, and macOS). It is more modular and lightweight compared to .NET Framework.
- Answer:
What is the Common Language Runtime (CLR)?
- Answer: The CLR is the runtime environment that executes .NET applications. It provides services like memory management, exception handling, garbage collection, and type safety. It compiles the Intermediate Language (IL) code into machine code.
What are value types and reference types in C#?
- Answer:
- Value types: Store data directly (e.g., int, char, double, struct). They are typically stored on the stack.
- Reference types: Store references to data (e.g., class, array, delegate). They are stored on the heap.
- Answer:
What is garbage collection in .NET?
- Answer: Garbage Collection (GC) is an automatic memory management feature in .NET. It frees up memory by removing objects that are no longer being used, preventing memory leaks.
What is boxing and unboxing in C#?
- Answer:
- Boxing: Converting a value type to a reference type (e.g., int to object).
- Unboxing: Converting a reference type back to a value type.
- Answer:
What is an assembly in .NET?
- Answer: An assembly is a compiled code library that contains metadata about the code and can be executed by the CLR. It is the basic unit of deployment in .NET, usually in the form of a .dll or .exe file.
What is the difference between a DLL and an EXE in .NET?
- Answer:
- DLL (Dynamic Link Library): Contains reusable code and is not directly executable.
- EXE (Executable File): A program that can be run as an application.
- Answer:
What is Just-In-Time (JIT) Compilation?
- Answer: JIT Compilation is the process where the CLR compiles Intermediate Language (IL) code into native machine code just before it is executed, improving performance by compiling only the needed code.
What is the Global Assembly Cache (GAC)?
- Answer: The GAC is a central repository for storing assemblies that are shared across multiple applications. It ensures that only one version of an assembly is loaded and used by different applications.
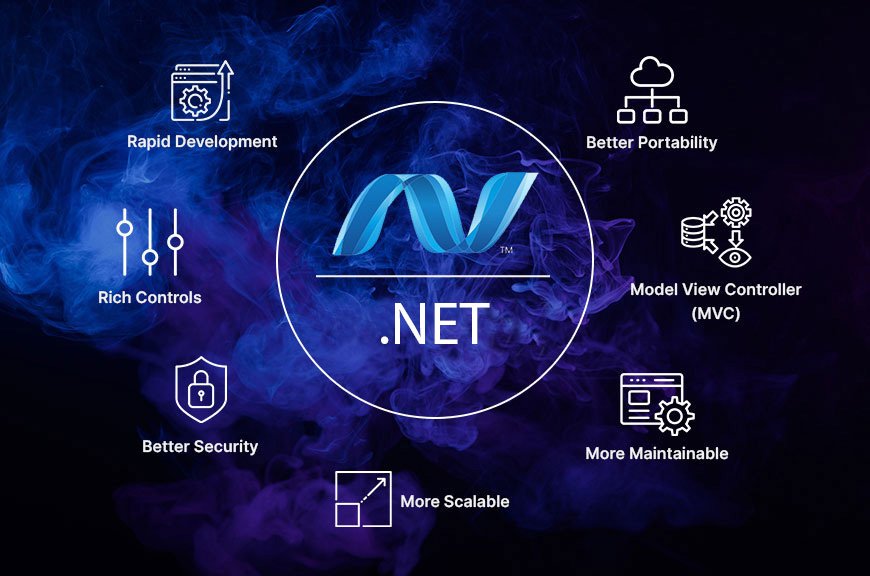
C# Programming Concepts
What is a class and an object in C#?
- Answer:
- Class: A blueprint or template for creating objects, defining properties and methods.
- Object: An instance of a class containing data and behavior.
- Answer:
What is encapsulation in C#?
- Answer: Encapsulation is the practice of restricting direct access to an object’s data and providing controlled access through public methods or properties, thereby protecting the integrity of the data.
What is inheritance in C#?
- Answer: Inheritance allows a class to inherit the members (fields, methods, etc.) of another class, enabling code reuse and the creation of hierarchical relationships. For example, a Car class can inherit from a Vehicle class.
What is polymorphism in C#?
- Answer: Polymorphism allows objects of different classes to be treated as objects of a common base class. It supports method overriding (runtime polymorphism) and method overloading (compile-time polymorphism).
What is the difference between method overloading and method overriding?
- Answer:
- Method overloading: Defining multiple methods with the same name but different parameter types or signatures.
- Method overriding: Redefining a method in a derived class that was already defined in the base class.
- Answer:
What is the static keyword in C#?
- Answer: The static keyword indicates that a member belongs to the type itself, rather than instances of the type. It means that the member is shared by all instances of the class.
What are delegates in C#?
- Answer: A delegate is a type-safe function pointer that can hold references to methods with a particular signature. It is commonly used in event handling and callback methods.
What is an event in C#?
- Answer: An event is a message sent by an object to signal the occurrence of an action. Events are typically used with delegates to allow the subscriber classes to handle the event when it is raised.
What is the difference between String and StringBuilder in C#?
- Answer:
- String: Immutable, meaning each modification creates a new object.
- StringBuilder: Mutable, meaning it allows modifications without creating new instances, making it more efficient for operations involving repeated changes to a string.
- Answer:
What are ref and out parameters in C#?
- Answer:
- ref: Requires the parameter to be initialized before it is passed to a method.
- out: The parameter doesn’t need to be initialized before passing, but the method must assign a value before returning.
- Answer:
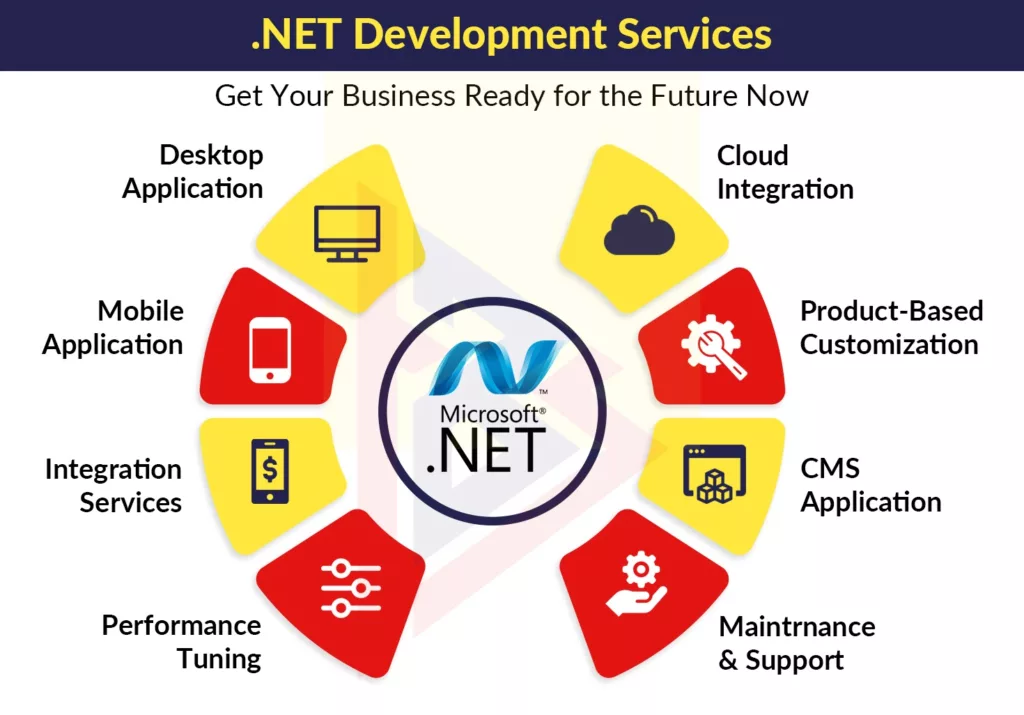
ASP.NET & Web Development Concepts
What is ASP.NET?
- Answer: ASP.NET is a web development framework for building dynamic web applications and services. It includes libraries for web forms, MVC (Model-View-Controller), and Web API development.
What is the difference between ASP.NET Web Forms and ASP.NET MVC?
- Answer:
- Web Forms: Uses event-driven programming with a drag-and-drop interface for rapid development.
- MVC: Follows the Model-View-Controller pattern, separating the business logic, user interface, and user input.
- Answer:
What is Web API in ASP.NET?
- Answer: Web API is a framework used to build HTTP-based services that can be consumed by various clients, such as browsers, mobile apps, and other web services.
What is routing in ASP.NET MVC?
- Answer: Routing is the mechanism that maps URLs to controller actions in ASP.NET MVC. It defines how a URL pattern should be matched to a specific controller and action.
What is ViewState in ASP.NET?
- Answer: ViewState is a mechanism in ASP.NET Web Forms that maintains the state of a page across HTTP requests. It stores the values of page controls to retain their state between requests.
What is the difference between Session and Cookies in ASP.NET?
- Answer:
- Session: Stores data on the server for a specific user session.
- Cookies: Stores small pieces of data on the client-side (browser) and can be accessed across multiple requests.
- Answer:
What is a Web Service in ASP.NET?
- Answer: A Web Service is a software system designed to support interoperable machine-to-machine interaction over a network. ASP.NET supports SOAP-based web services.
What is the role of the Global.asax file in ASP.NET?
- Answer: The Global.asax file, also known as the application file, is used for handling application-level events like Application_Start, Application_End, and Session_Start.
What is a controller in ASP.NET MVC?
- Answer: A controller is a class in ASP.NET MVC that handles incoming requests, processes the business logic, and returns the response in the form of views or data.
What is Dependency Injection (DI)?
- Answer: DI is a design pattern that allows objects to be passed their dependencies rather than creating them internally, facilitating loose coupling and easier testing.
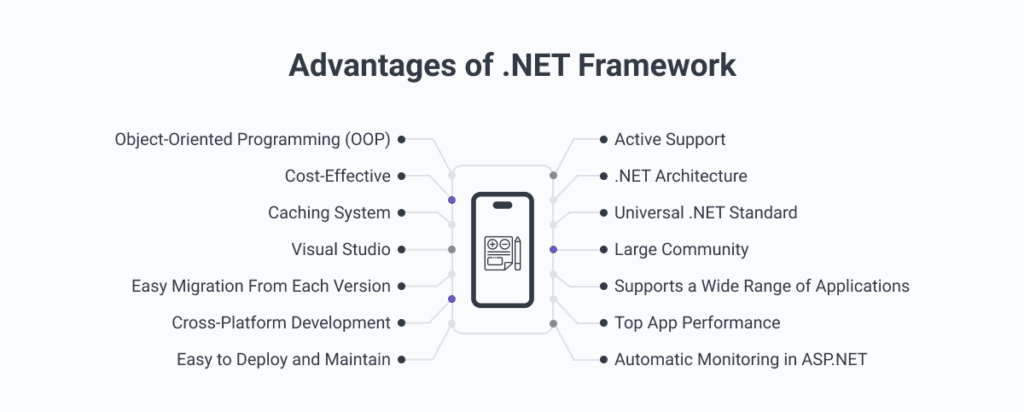
Advanced .NET Concepts
What is ADO.NET?
- Answer: ADO.NET is a set of classes in .NET for data access. It provides a way to interact with databases using objects like Connection, Command, DataReader, and DataAdapter.
What is Entity Framework?
- Answer: Entity Framework (EF) is an Object-Relational Mapping (ORM) framework for .NET that allows developers to work with relational data as objects, eliminating the need for most SQL queries.
What is the difference between ADO.NET and Entity Framework?
- Answer:
- ADO.NET: Provides a lower-level approach to data access, requiring SQL queries to retrieve and manipulate data.
- Entity Framework: A higher-level ORM framework that abstracts database access by mapping entities to tables in the database.
- Answer:
What are LINQ (Language Integrated Query) and its types?
- Answer: LINQ is a feature that allows querying collections (arrays, lists, or databases) using C# syntax. Types of LINQ include LINQ to Objects, LINQ to SQL, LINQ to Entities, and LINQ to XML.
What is WCF (Windows Communication Foundation)?
- Answer: WCF is a framework for building service-oriented applications. It allows communication between client and server applications over different protocols (HTTP, TCP, etc.).
What is SignalR in ASP.NET?
- Answer: SignalR is a library for adding real-time web functionality to ASP.NET applications. It enables bi-directional communication between server and client, commonly used for chat applications, live notifications, etc.
What is multi-threading in .NET?
- Answer: Multi-threading allows the execution of multiple threads (smaller units of a process) in parallel, improving performance for tasks like background processing or UI responsiveness.
What are background tasks in ASP.NET?
- Answer: Background tasks in ASP.NET can be executed asynchronously, typically for tasks such as sending emails, processing data, or handling time-consuming operations.
What is the role of the async and await keywords in C#?
- Answer:
- async: Marks a method as asynchronous, allowing it to run asynchronously without blocking the calling thread.
- await: Used inside an async method to indicate where the program should wait for a task to complete before continuing.
- Answer:
What is the Singleton design pattern in C#?
- Answer: The Singleton pattern ensures that a class has only one instance throughout the application and provides a global point of access to it. This is useful for managing resources like database connections.
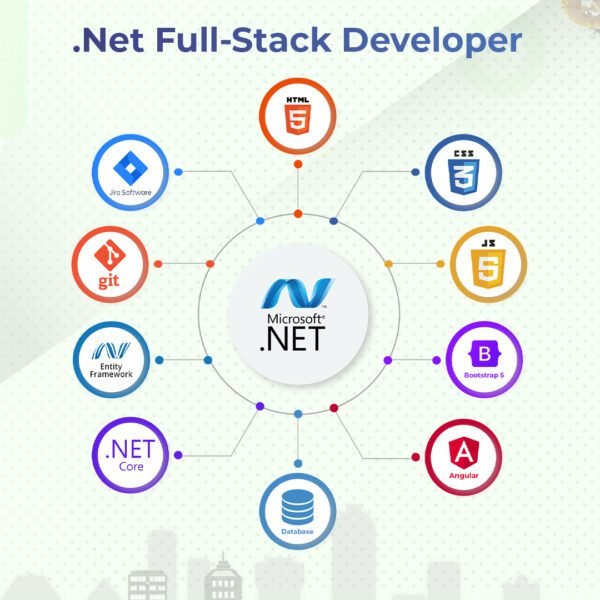
These answers will help freshers understand key .NET concepts and prepare for interviews confidently.