Table of Contents
ToggleHow to Learn Java Programming Step by Step for Beginners
Here’s a polished version of the step-by-step guide titled “How to Learn Java Programming Step by Step for Beginners” with added clarity, simplicity, and a user-friendly structure:
How to Learn Java Programming Step by Step for Beginners
Java is a powerful and versatile programming language widely used in software development. For beginners, learning Java can open doors to careers in web development, mobile applications, and enterprise software. Follow this step-by-step guide to master Java programming effectively.
Step 1: Understand Programming Fundamentals
Start by familiarizing yourself with essential programming concepts, such as:
- Variables: Storage for data (e.g., integers, strings).
- Data Types: Types of data (e.g., int, float, char).
- Loops: Repeated execution of code blocks (for, while).
- Conditionals: Decision-making using if-else.
Example: Writing Your First Java Program
java
Copy code
public class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
Step 2: Set Up Your Development Environment
To start coding in Java, you need the right tools:
- Install the JDK (Java Development Kit): Download it from Oracle’s official site.
- Set up an IDE: Use beginner-friendly IDEs like IntelliJ IDEA, Eclipse, or NetBeans.
Tip: Follow official setup guides to configure your environment properly.
Step 3: Learn Core Java Syntax
Understand Java’s syntax and write simple programs using:
- If-Else Statements: Make decisions in your code.
- Loops: Automate repetitive tasks.
- Arrays: Store multiple values in a single variable.
Exercise: Write a program to print the multiplication table of 5.
java
Copy code
public class MultiplicationTable {
public static void main(String[] args) {
int num = 5;
for (int i = 1; i <= 10; i++) {
System.out.println(num + ” x ” + i + ” = ” + (num * i));
}
}
}
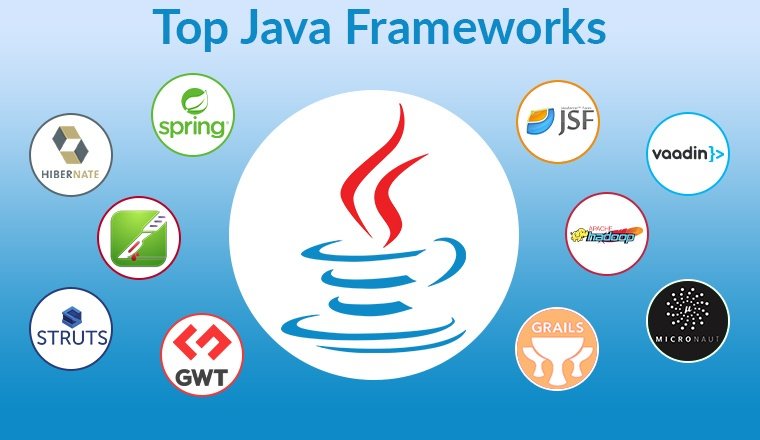
Step 4: Master Object-Oriented Programming (OOP)
OOP is a fundamental concept in Java. Learn these principles:
- Classes and Objects: Blueprint and instances.
- Inheritance: Reuse code across classes.
- Polymorphism: Use a single interface for different types.
- Encapsulation: Keep data secure within classes.
Example:
java
Copy code
class Animal {
void sound() {
System.out.println(“This is an animal”);
}
}
class Dog extends Animal {
void sound() {
System.out.println(“Dog barks”);
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound();
}
}
Step 5: Practice Through Projects
Apply your knowledge with small, manageable projects:
- A Simple Calculator: Perform basic arithmetic operations.
- To-Do List Manager: Add, remove, and list tasks.
Why Projects Matter: They help solidify your understanding and prepare you for real-world applications.
Step 6: Debugging and Testing
Learn how to identify and fix errors:
- Debugging Tools: Use features in your IDE to inspect code behavior.
- Unit Testing: Write test cases for small code segments.
Example: Use try-catch blocks to handle exceptions.
java
Copy code
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println(“Cannot divide by zero!”);
}
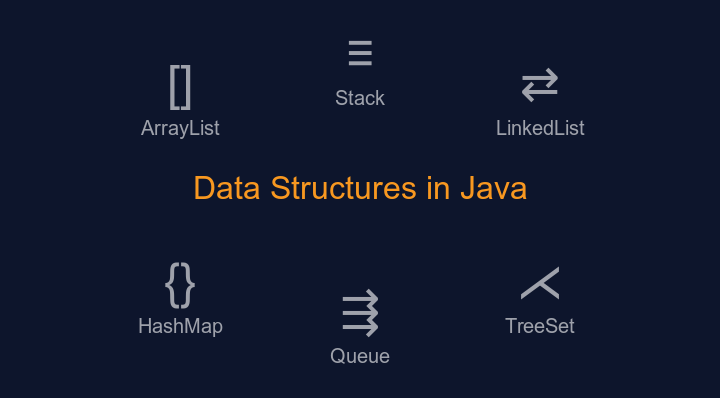
Step 7: Learn Advanced Concepts
Once comfortable with the basics, explore:
- Streams: Handle data processing efficiently.
- Collections Framework: Work with lists, maps, and sets.
- Exception Handling: Manage runtime errors gracefully.
Tip: Experiment with multithreading to understand concurrent programming.
Step 8: Build a Portfolio
Showcase your skills with projects that solve real-world problems:
- E-commerce Application: Allow users to browse products and make purchases.
- Library Management System: Track books and user activities.
Why Portfolio Projects Are Important: They demonstrate your abilities to potential employers.
Step 9: Join a Community
Stay motivated by connecting with others:
- Forums: Join communities like Stack Overflow or Reddit.
- GitHub: Share your projects and contribute to others’ work.
- Local Meetups: Engage with local developer groups.
Networking Benefit: Learn from experienced developers and get career guidance.
Step 10: Keep Learning
Java has an extensive ecosystem. Explore:
- Frameworks: Learn Spring for building web applications and Hibernate for database management.
- Microservices: Understand how to build scalable, distributed systems.
- Certifications: Pursue certifications like Oracle’s Java SE Programmer to validate your skills.
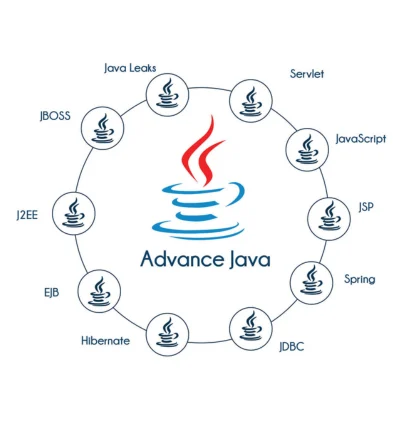
Final Tips
- Be Consistent: Practice coding daily.
- Start Small: Focus on simple tasks before tackling advanced projects.
- Seek Feedback: Share your work with peers or mentors for improvement.