Table of Contents
ToggleDATA STRUCTURES AND ALGORITHMS
Mastering data structures and algorithms (DSA) is a critical skill for any aspiring software engineer, data scientist, or computer scientist. A solid understanding of DSA not only enhances your problem-solving abilities but also prepares you for technical interviews and complex programming tasks. Here’s a comprehensive guide on how to effectively master DSA.
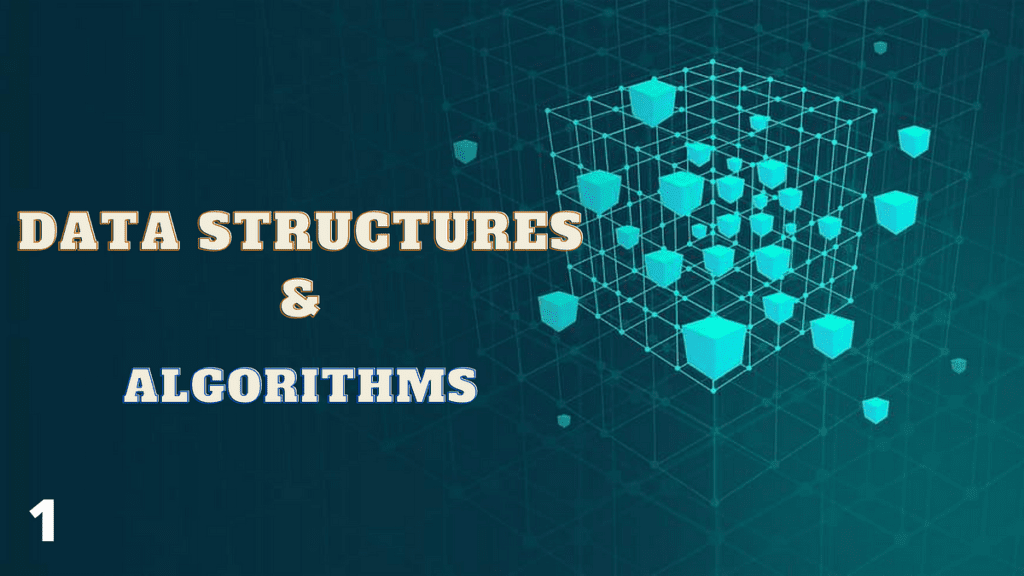
Understanding the Fundamentals
Before diving into complex topics, it’s essential to grasp the foundational concepts of data structures and algorithms. Begin by getting acquainted with the fundamental data structures:
Arrays: Simple collections of elements stored in contiguous memory. Understand their operations (insertion, deletion, access) and limitations (fixed size, costly insertions/deletions).
Linked Lists: A series of nodes where each node points to the next Study singly linked lists, doubly linked lists, and circular linked lists. Investigate singly linked lists, doubly linked lists, and circular linked lists.
Stacks: LIFO (Last In, First Out) structures used for tasks like function calls and undo mechanisms.
Queues: FIFO (First In, First Out) structures used in scenarios like scheduling tasks and handling requests.
Trees: Hierarchical data structures like binary trees, binary search trees, AVL trees, and heaps, which are used for efficient data retrieval and storage.
Graphs: Collections of vertices and edges. Understand directed vs. undirected graphs and weighted vs. unweighted graphs.
Familiarizing yourself with these structures lays the groundwork for more advanced topics.
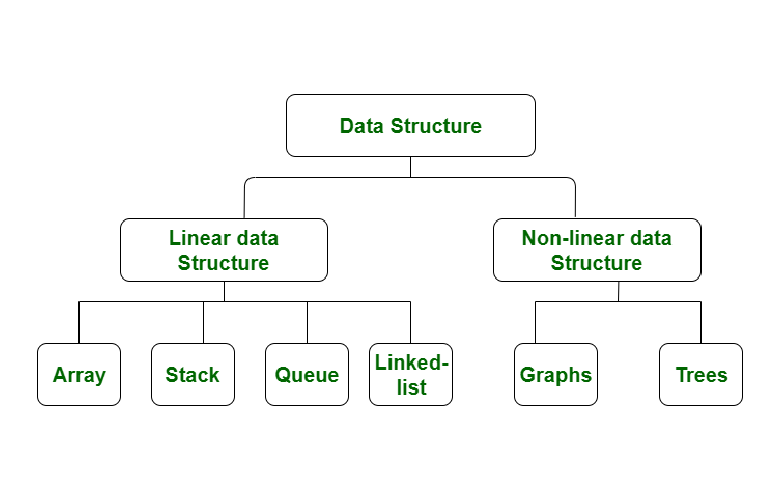
Learn Algorithms
Once you have a grasp of data structures, move on to studying algorithms. Start with basic algorithms and progressively tackle more complex ones:
Sorting Algorithms: Learn different methods like bubble sort, selection sort, insertion sort, merge sort, and quicksort. Understanding the time complexity and space complexity of these algorithms is crucial.
Searching Algorithms: Familiarize yourself with linear search and binary search, and understand when to use each based on the data structure.
Graph Algorithms: Study algorithms such as breadth-first search (BFS), depth-first search (DFS), Dijkstra’s algorithm, and the A* algorithm for pathfinding.
Dynamic Programming: Explore problems that can be broken down into smaller, overlapping subproblems, such as the Fibonacci sequence and the knapsack problem.
Select Learning Resources
Selecting the appropriate resources can greatly enhance your learning experience.
Books: Some classic texts include “Introduction to Algorithms” by Cormen et al., which provides in-depth coverage of various algorithms, and “Data Structures and Algorithms Made Easy” by Narasimha Karumanchi for practical insights.
YouTube: Many channels provide excellent tutorials and explanations of algorithms and data structures, making it easier to grasp complex concepts.
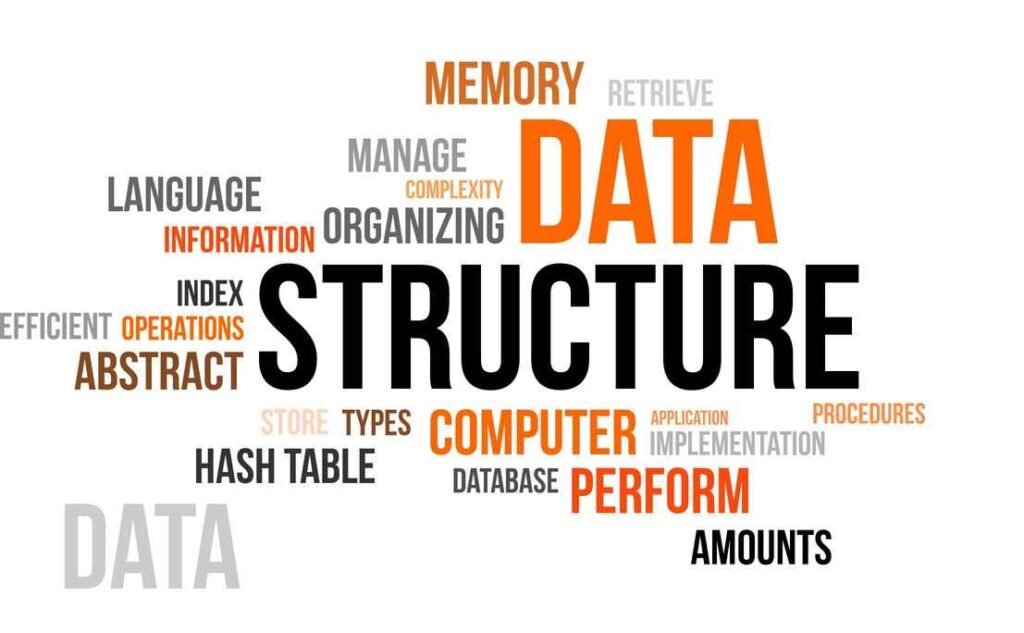
Consistent Practice
Consistent practice is key to mastering DSA:
Coding Platforms: Utilize websites like LeetCode, HackerRank, CodeSignal, and Codewars to solve a variety of problems. Start with easy problems and gradually move to medium and hard levels.
Daily Challenges: Aim to solve a specific number of problems each week. This will help reinforce your learning and improve your coding speed.
Mock Interviews: Engage in timed mock interviews to simulate real interview conditions. Platforms like Pramp and Interviewing.io offer practice with peers or mentors.
Implement Projects
Applying your knowledge through projects can deepen your understanding of DSA:
Real-World Applications: Build projects that utilize various data structures and algorithms, such as a personal library system, a simple game, or a task manager. This hands-on experience will reinforce your theoretical knowledge.
Open Source Contribution: Participate in open-source projects to see how DSA is used in real-world applications. This can provide exposure to collaborative coding and project management.
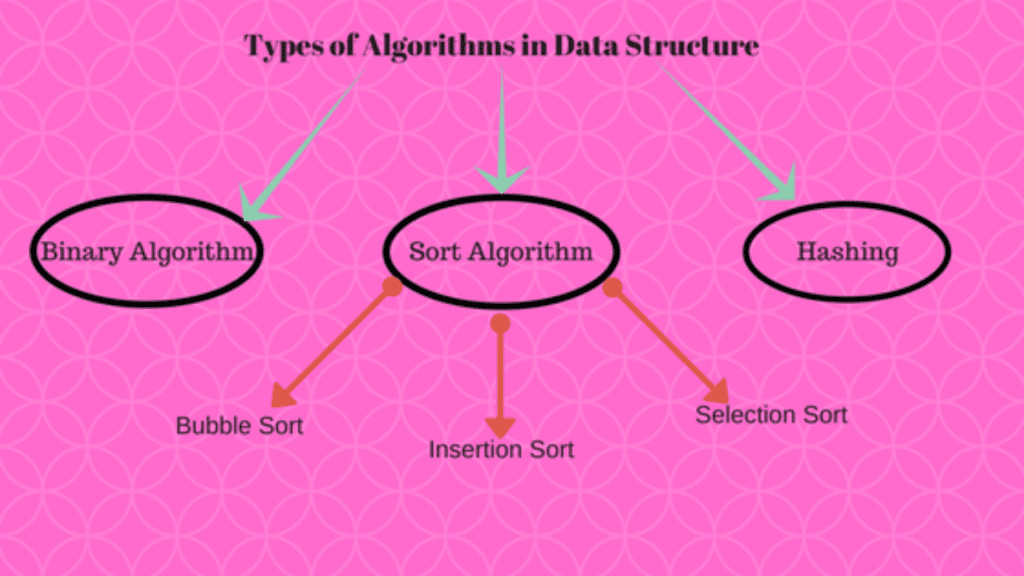
Understand Time and Space Complexity
Understanding algorithm efficiency is crucial:
Big O Notation: Understand how to evaluate the time and space complexity of algorithms using Big O notation.
Optimize Solutions: As you solve problems, always think about how you can optimize your solution. This might involve choosing a different data structure or a more efficient algorithm.
Engage with the Community
Joining a community can provide support and resources:
Online Forums: Participate in forums like Stack Overflow, Reddit (subreddits like r/learnprogramming), and Discord groups focused on programming. Engage in discussions, ask questions, and share knowledge.
Local Meetups: Look for local coding groups or meetups where you can collaborate with others, share insights, and learn from each other.
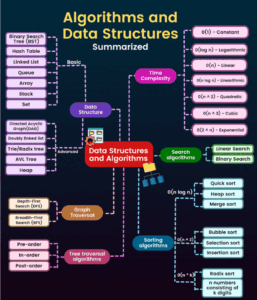
Review and Reflect
Regular review is essential for retention:
Revisit Concepts: Periodically go back to concepts you’ve already learned. Teaching others or explaining concepts to a peer can reinforce your understanding.
Analyze Mistakes: When you struggle with a problem, analyze what went wrong and how you can improve. Learning from mistakes is a crucial part of the process.
Stay Committed and Patient
Mastery takes time:
Set Realistic Goals: Establish short- and long-term goals for your learning journey. Divide larger topics into smaller, manageable sections to prevent feeling overwhelmed.
Foster a Growth Mindset: Welcome challenges and see setbacks as chances to learn.
Stay Consistent and Patient
Mastery takes time. Stay motivated and keep a growth mindset. Celebrate small victories along the way!
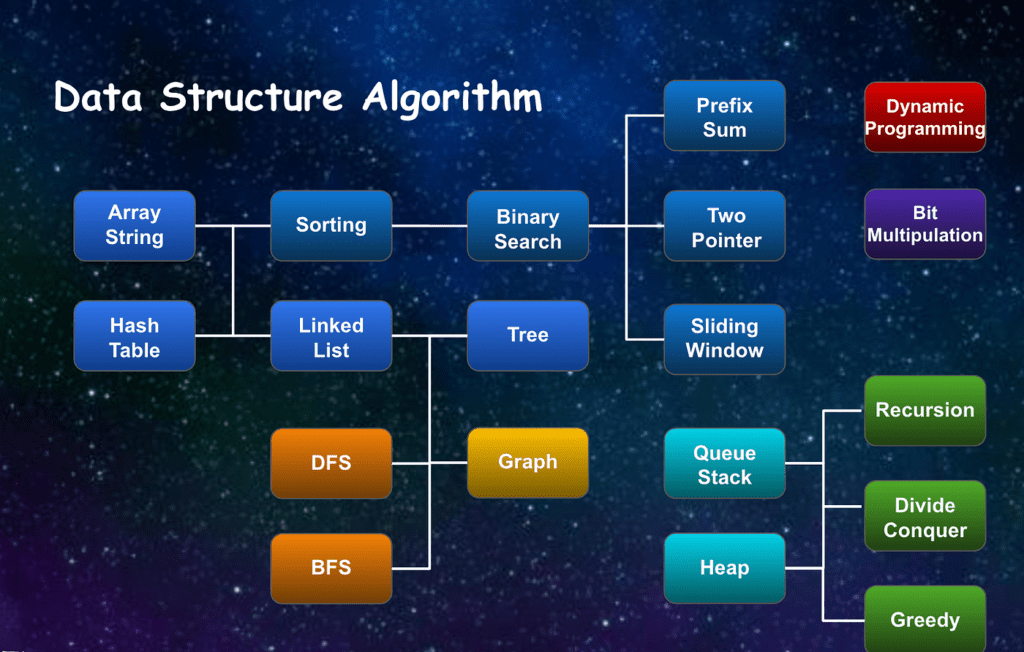
Final Tips:
Decompose Problems: Practice dividing complex issues into manageable components.
Share Your Knowledge: Teaching concepts to others can strengthen your own understanding.
Stay Updated: DSA is an evolving field; keep learning about new algorithms and best practices.
Conclusion
Mastering data structures and algorithms is a journey that combines theoretical knowledge with practical application. By building a strong foundation, practicing consistently, and engaging with the community, you’ll develop the skills necessary to tackle complex programming challenges and excel in technical interviews. Stay committed, keep learning, and enjoy the process!