Table of Contents
ToggleJAVA INHERITANCE INTERVIEW QUESTIONS
Here’s a detailed list of 25 important interview questions about Java inheritance, along with descriptions for each:
What is inheritance in Java?
Inheritance is a fundamental concept of object-oriented programming that allows a class (subclass) to inherit properties and methods from another class (superclass), promoting code reuse and establishing a relationship between classes.
What are the types of inheritance supported in Java?
Java supports single inheritance (one subclass extends one superclass), multilevel inheritance (a subclass extends another subclass), and hierarchical inheritance (multiple subclasses extend a single superclass). Java does not allow multiple inheritance with classes to prevent complexity and ambiguity.
How do you implement inheritance in Java?
Inheritance is implemented using the `extends` keyword. For example:
“`java
class Animal { }
class Dog extends Animal { }
“`
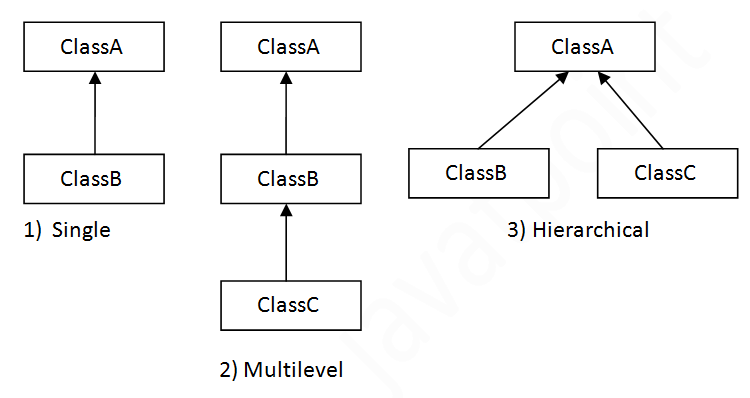
What is the `super` keyword used for?
The `super` keyword is used to refer to the immediate superclass of a class. It can be used to access superclass methods and constructors, allowing subclasses to utilize inherited features.
Can a subclass call a superclass constructor?
Yes, a subclass can call a superclass constructor using the `super()` keyword. If not explicitly called, the default constructor of the superclass is invoked automatically.
What happens if a subclass does not explicitly call a superclass constructor?
If a subclass does not call a superclass constructor, Java automatically calls the no-argument constructor of the superclass. If no such constructor exists, a compilation error occurs.
What is method overriding?
Method overriding happens when a subclass offers a specific implementation of a method that has already been defined in its superclass, enabling dynamic method dispatch.
What is the `@Override` annotation?
The `@Override` annotation indicates that a method is intended to override a method in the superclass. It helps identify errors at compile time if the method does not correctly override a superclass method.
What distinguishes method overloading from method overriding?
Method overloading occurs within a single class and involves multiple methods that share the same name but have different parameters. Method overriding occurs between a superclass and subclass, allowing the subclass to provide a specific implementation of a superclass method.
Can a subclass override a private method from its superclass?
No, a private method in a superclass is not accessible to subclasses, and therefore, cannot be overridden.
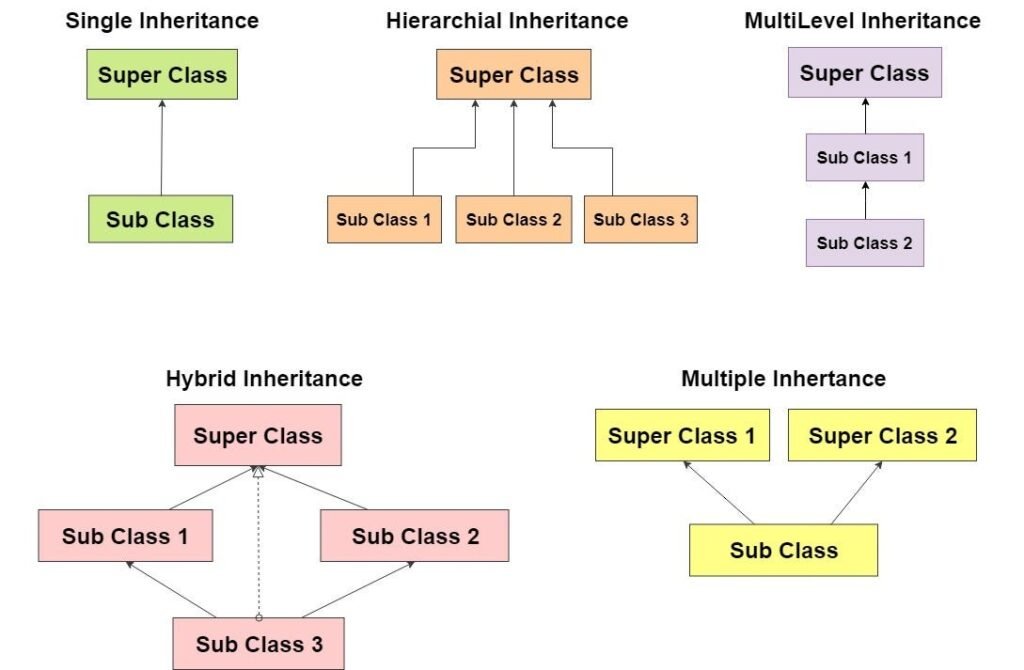
What are abstract classes and how are they related to inheritance?
An abstract class cannot be instantiated and may contain abstract methods (without implementations) that must be implemented by subclasses. They provide a way to define a common base class with shared functionality.
Can a class inherit multiple interfaces?
Yes, a class can implement multiple interfaces, allowing it to inherit behaviors from several sources, which is a way to achieve multiple inheritance in Java.
What is the diamond problem in inheritance?
The diamond problem arises when a class inherits from two classes that have a common ancestor, leading to ambiguity in method resolution. Java prevents this by disallowing multiple inheritance with classes.
How can you prevent a class from being inherited?
A class can be declared as `final` to prevent it from being subclassed. This is useful when a complete and unmodifiable implementation is required.
What are covariant return types?
Covariant return types allow a subclass to override a method and return a type that is a subclass of the return type declared in the superclass method. This enhances flexibility in method overriding.
What is the role of the `Object` class in Java inheritance?
The `Object` class acts as the foundation of the class hierarchy in Java. Every class inherits from `Object`, which provides basic methods (like `toString()`, `equals()`, and `hashCode()`) that can be overridden by subclasses.
How do static methods behave in inheritance?
Static methods are associated with the class itself rather than with its instances. They cannot be overridden but can be hidden if a subclass defines a static method with the same name.
What happens if you override a static method in a subclass?
If a static method in a subclass has the same name as a static method in the superclass, it hides the superclass method. The method that gets called is determined by the reference type, not the object type.
Can an interface extend another interface?
Yes, an interface can extend another interface, enabling it to inherit method signatures from its parent interface.
What is the significance of the `default` keyword in interfaces?
The `default` keyword enables an interface to provide a default implementation for a method. This helps add new methods to interfaces without breaking existing implementations.
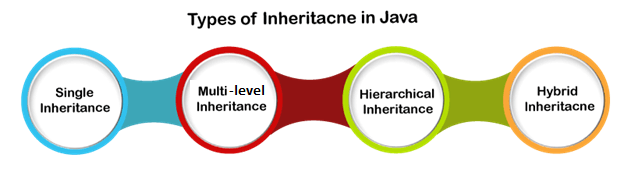
How does polymorphism relate to inheritance?
Polymorphism allows methods to be called on an object without knowing its specific type at compile time. Inheritance enables polymorphism through method overriding and interfaces.
What is the relationship between inheritance and code reuse?
Inheritance promotes code reuse by allowing subclasses to inherit and extend the behavior of their superclasses, reducing redundancy and improving maintainability.
Can constructors be inherited in Java?
No, constructors are not inherited. A subclass must define its own constructors and can call the superclass constructor using `super()`.
What are the best practices for using inheritance in Java?
Use inheritance to model “is-a” relationships, favor composition over inheritance when possible, avoid deep inheritance hierarchies, and ensure that subclasses are meaningful extensions of their superclasses.
How does Java handle method resolution in inheritance chains?
Java uses a specific order called the method resolution order (MRO) to determine which method to call. It first looks in the subclass, then in its superclass, and continues up the hierarchy until it finds the method or reaches `Object`.
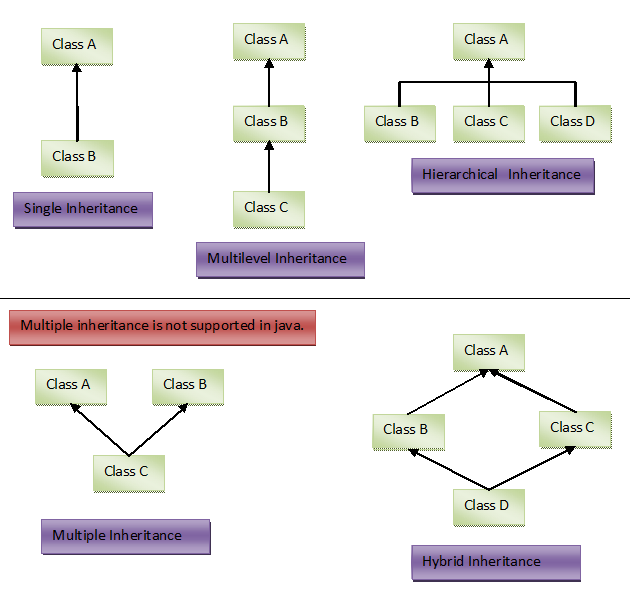
Conclusion
Java inheritance is a cornerstone of object-oriented programming, enabling developers to create well-structured, reusable, and maintainable code. Mastery of inheritance concepts—such as method overriding, the use of the `super` and `final` keywords, and understanding the roles of abstract classes and interfaces—is essential for effective software design.
Candidates preparing for interviews should be able to articulate these concepts clearly and provide practical examples, as these questions often assess not only knowledge but also the ability to apply that knowledge in real-world scenarios. A strong grasp of inheritance will not only aid in passing interviews but also in building robust Java applications.