Table of Contents
ToggleLWC interview questions in salesforce
Here are 30 important LWC interview questions for Salesforce developers, which cover key concepts, best practices, and common use cases:
What is Lightning Web Component (LWC)?
- Answer: LWC is a JavaScript framework that is built using web standards like HTML, CSS, and JavaScript, and it runs in the browser. It is used for developing fast, lightweight, and reusable components in Salesforce.
How is LWC different from Aura Components?
- Answer: LWC is based on modern web standards, is more lightweight, and offers better performance compared to Aura, which is built on Salesforce’s proprietary framework. LWC uses fewer resources and integrates more closely with native browser APIs.
What are the core features of LWC?
- Answer: Core features of LWC include:
- Based on modern JavaScript (ES6+).
- Better performance using native browser APIs.
- Reusable and encapsulated components.
- Built-in data binding and event handling.
- Integration with Salesforce data using wire adapters and Apex.
What are the lifecycle hooks in LWC?
- Answer: LWC has several lifecycle hooks:
- constructor()
- connectedCallback()
- disconnectedCallback()
- renderedCallback()
- errorCallback()
What is the @api decorator in LWC?
- Answer: @api is used to expose properties and methods of a component to its parent. It makes a property or method public, allowing it to be accessed by other components.
What is the @track decorator in LWC?
- Answer: The @track decorator is used to make an object or array reactive, meaning that when its properties change, the component re-renders. However, with modern LWC, reactivity is automatic for primitive values, and @track is only necessary for complex objects/arrays.
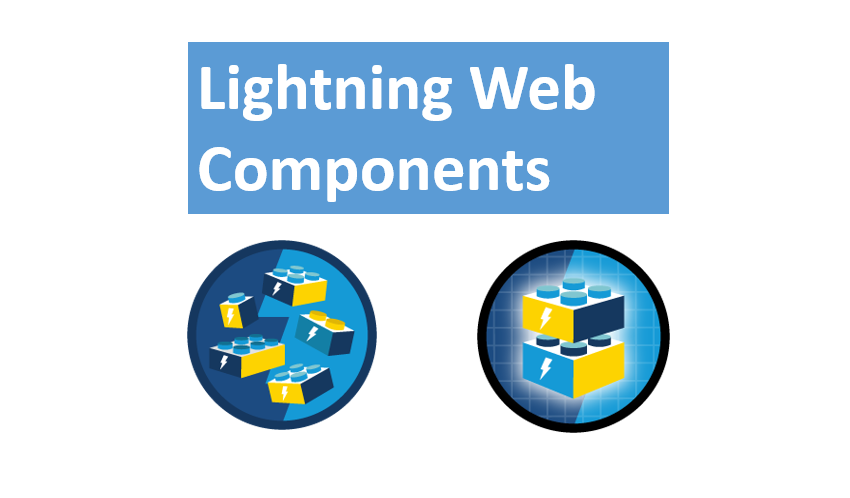
What is the purpose of @wire in LWC?
- Answer: The @wire decorator is used to read data from Salesforce servers or Apex methods and automatically update the component when the data changes. It allows for declarative data fetching and integration with Salesforce.
How do you pass data from a parent to a child component in LWC?
- Answer: Data is passed via public properties in the child component, which are decorated with the @api decorator. The parent component binds its data to these properties.
How do you pass data from a child to a parent component in LWC?
- Answer: Data is passed via custom events. The child component fires an event, and the parent listens for that event to retrieve the data.
What is Lightning Data Service (LDS) in LWC?
- Answer: LDS is a declarative way to work with Salesforce records without needing to write Apex. It handles record caching, sharing rules, and field-level security. LDS is often used with the @wire decorator for data retrieval.
What is the LightningElement class in LWC?
- Answer: LightningElement is the base class for all LWC components. It provides methods like render(), connectedCallback(), disconnectedCallback(), and other lifecycle hooks.
Can you call Apex methods from LWC?
- Answer: Yes, Apex methods can be called from LWC using the @wire decorator or imperatively. The @wire decorator is for declarative calls, and imperatively, Apex methods can be invoked with promises.
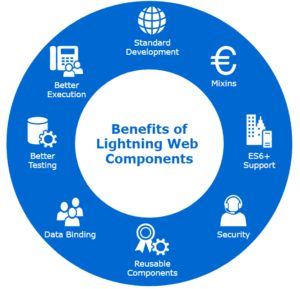
What is Locker Service, and how does it affect LWC?
- Answer: Locker Service is a security feature that isolates components to prevent access to other components’ data. It enforces strict security policies, such as restricting access to global JavaScript objects and enforcing best practices for security.
How do you handle errors in LWC?
- Answer: Errors can be handled using the errorCallback() lifecycle hook, or you can handle errors in Apex methods by using catch() in promise chains.
How do you optimize LWC performance?
- Answer: Performance can be optimized by:
- Reducing unnecessary re-renders.
- Using wire services for efficient data fetching.
- Minimizing the use of complex object tracking.
- Using async operations and avoiding blocking the main thread.
What are the differences between @wire and imperative Apex calls in LWC?
- Answer:
- @wire: Automatically calls Apex and binds the result to a property. Re-renders the component when data changes.
- Imperative Apex calls: Manually call Apex methods using promises, giving more control over when to execute and how to handle the result.
How do you test LWC components?
- Answer: LWC components can be tested using Jest, a testing framework supported by Salesforce. You can mock Apex calls and simulate DOM manipulation to ensure components work as expected.
What is the shadow DOM in LWC?
- Answer: The Shadow DOM in LWC is used to encapsulate the component’s structure and styling. It prevents styles from leaking out to other components and ensures that the component’s internal styles do not affect other parts of the application.
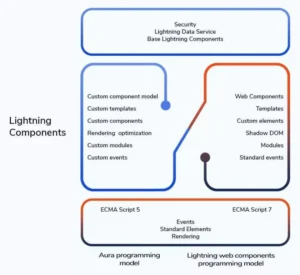
How do you import static resources into LWC?
- Answer: Static resources can be imported using the @salesforce/resourceUrl module. For example:
js
Copy code
import myResource from ‘@salesforce/resourceUrl/myStaticResource’;
Can you use JavaScript frameworks like React or Angular with LWC?
- Answer: No, LWC cannot directly use other JavaScript frameworks like React or Angular due to its strict use of the native browser APIs and Salesforce’s Locker Service restrictions.
How do you handle styles in LWC?
- Answer: LWC styles are scoped to the component, ensuring that the styles do not affect other components. Styles can be written in a .css file, and Salesforce Lightning Design System (SLDS) can be used for consistent design.
What is a custom event in LWC, and how do you fire one?
- Answer: A custom event allows one component to communicate with another by firing an event. The event is fired using this.dispatchEvent(event) in the child component. The parent listens to the event and handles it.
What are reactive properties in LWC?
- Answer: Reactive properties are variables that automatically trigger a re-render of the component when their value changes. In LWC, reactivity is automatic for primitive types, and objects or arrays are reactive by default if they are tracked.
What are the common challenges in LWC development?
- Answer: Common challenges include:
- Dealing with Locker Service restrictions.
- Managing component state efficiently.
- Understanding the reactivity system and optimizing performance.
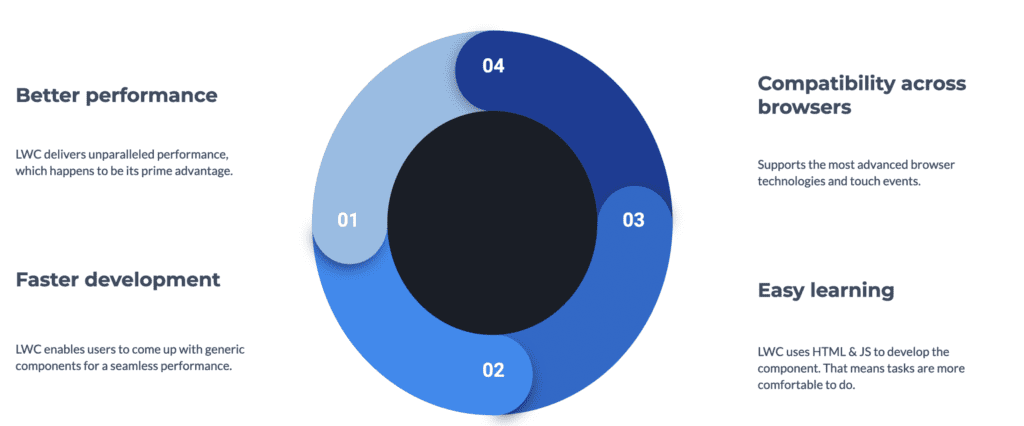
How do you use wire adapters in LWC?
- Answer: Wire adapters are used to retrieve data from Salesforce or Apex methods. For example:
js
Copy code
@wire(getRecord, { recordId: ‘$recordId’, fields: [NAME_FIELD] })
record;
How do you handle pagination in LWC when fetching large datasets?
- Answer: Pagination can be implemented by using @wire with parameters to load smaller chunks of data, such as offset and limit. Alternatively, Apex can handle pagination logic and return smaller data sets.
What is renderedCallback() in LWC?
- Answer: renderedCallback() is called after every render of the component. It is used when you need to interact with the DOM after the component has been rendered, such as manipulating third-party libraries or performing DOM operations.
What is @wire used for in Apex calls?
- Answer: @wire is used to automatically fetch data from Apex methods or Salesforce data services. The result is automatically wired to a property or method and re-renders the component when the data changes.
Can you use a third-party JavaScript library in LWC?
- Answer: Yes, third-party JavaScript libraries can be used by importing them as static resources and then loading them inside the LWC component.
What is connectedCallback() used for in LWC?
- Answer: connectedCallback() is called when a component is inserted into the DOM. It is commonly used for initialization tasks, such as fetching data or setting up event listeners.
Conclusion
These 30 questions are fundamental for any Salesforce developer working with Lightning Web Components (LWC). LWC offers numerous advantages, including modern web standards, better performance, and reusable components. However, it also comes with certain complexities like understanding the lifecycle, handling reactivity, and managing security with Locker Service. By mastering LWC, Salesforce developers can create highly efficient, scalable, and future-proof applications on the Salesforce platform.