Table of Contents
ToggleNode.js Full Course for Beginners
Node.js is not a programming language; it is a runtime environment that enables you to execute JavaScript on the server side.enabling the development of scalable, fast, and real-time applications. This course will take you through the basics of Node.js, including how to set it up, its core features, and how to build a simple web application.
Here’s a comprehensive outline for a Node.js full course for beginners. This course will cover the fundamentals of Node.js, from setting up the environment to building real-world applications.
Introduction to Node.js
Node.js is a runtime environment, not a programming language, that enables you to execute JavaScript on the server.Traditionally, JavaScript was used only for front-end development in browsers With Node.js, you can now build full-stack applications, using JavaScript for both the front-end and back-end.
What is Node.js?
- JavaScript runtime built on Chrome’s V8 JavaScript engine.
- It allows running JavaScript on the server side.
- Non-blocking, event-driven architecture for scalable applications.
- Why use Node.js?
- Real-time applications (e.g., chat apps, online gaming).
- Efficient handling of I/O-bound tasks.
- Single language for front-end (JavaScript) and back-end (Node.js).
- Installing Node.js
- Install Node.js from the official website (https://nodejs.org/).
- Verify installation by running node -v and npm -v in the terminal.
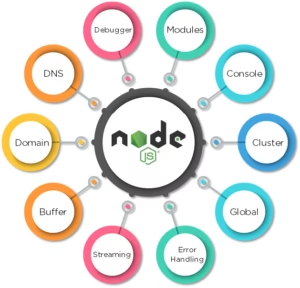
Basics of Node.js
- Creating a Simple Node.js Server
js
Copy code
const http = require(‘http’);
const server = http.createServer((req, res) => {
res.write(‘Hello World’);
res.end();
});
server.listen(3000, () => {
console.log(‘Server is running on port 3000’);
});
- The Event Loop
- Understanding the asynchronous nature of Node.js.
- Callbacks, Promises, and Async/Await.
Understanding NPM (Node Package Manager)
- What is NPM?
- NPM is the default package manager for Node.js.
- Using NPM to Install Packages
- Initialize a project with npm init.
- Install a package: npm install <package-name>.
- Understanding package.json
- Dependency management.
- Scripts in package.json.
json
Copy code
{
“name”: “nodejs-course”,
“version”: “1.0.0”,
“scripts”: {
“start”: “node app.js”
},
“dependencies”: {
“express”: “^4.17.1”
}
}
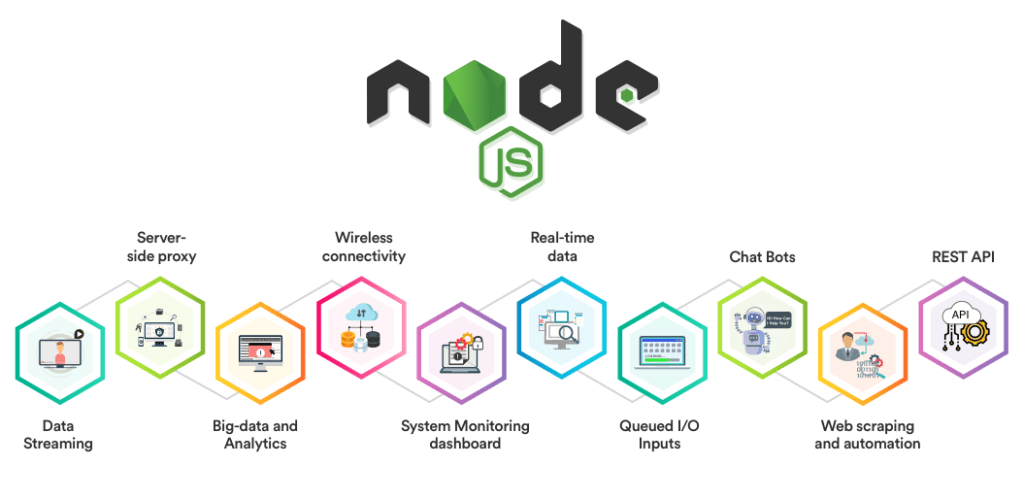
Working with Modules
- Built-in Modules
- HTTP, File System (fs), Path, OS, etc.
- Example: Reading a file using the fs module.
js
Copy code
const fs = require(‘fs’);
fs.readFile(‘example.txt’, ‘utf8’, (err, data) => {
if (err) throw err;
console.log(data);
});
- Creating Custom Modules
- module.exports and require to share functionality between files.
Express.js: Web Framework for Node.js
- What is Express?
- Fast and minimalist web framework for Node.js.
- Setting Up an Express Application
js
Copy code
const express = require(‘express’);
const app = express();
app.get(‘/’, (req, res) => {
res.send(‘Hello, Express!’);
});
app.listen(3000, () => {
console.log(‘Server is running on port 3000’);
});
- Routing and Middleware
- Handling different HTTP methods (GET, POST, PUT, DELETE).
- Using middleware for logging, authentication, etc.
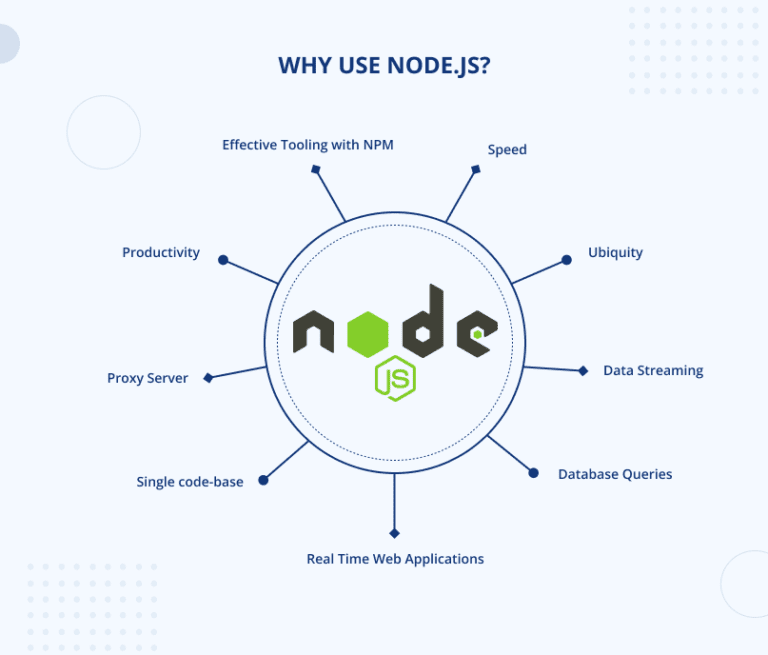
Working with Databases
- MongoDB with Mongoose
- Introduction to NoSQL and MongoDB.
- Install MongoDB and Mongoose for database interaction.
- Example: Connect to MongoDB and perform CRUD operations.
js
Copy code
const mongoose = require(‘mongoose’);
mongoose.connect(‘mongodb://localhost/test’, { useNewUrlParser: true, useUnifiedTopology: true });
const User = mongoose.model(‘User’, { name: String, age: Number });
const user = new User({ name: ‘John Doe’, age: 30 });
user.save().then(() => console.log(‘User saved’));
- Using MySQL or PostgreSQL (Optional)
- Introduction to SQL databases.
- Install packages like mysql2 or pg for database interaction.
Authentication and Authorization
- JWT (JSON Web Tokens) Authentication
- Secure user authentication using JWT tokens.
- Example: User login and token generation.
- Passport.js for Authentication
- Integrating third-party authentication systems (e.g., Google, Facebook).
Asynchronous Programming in Node.js
- Callbacks
- Handling asynchronous operations with callbacks.
- Promises
- Using promises to avoid callback hell.
- Async/Await
- Simplifying asynchronous code with async/await syntax.
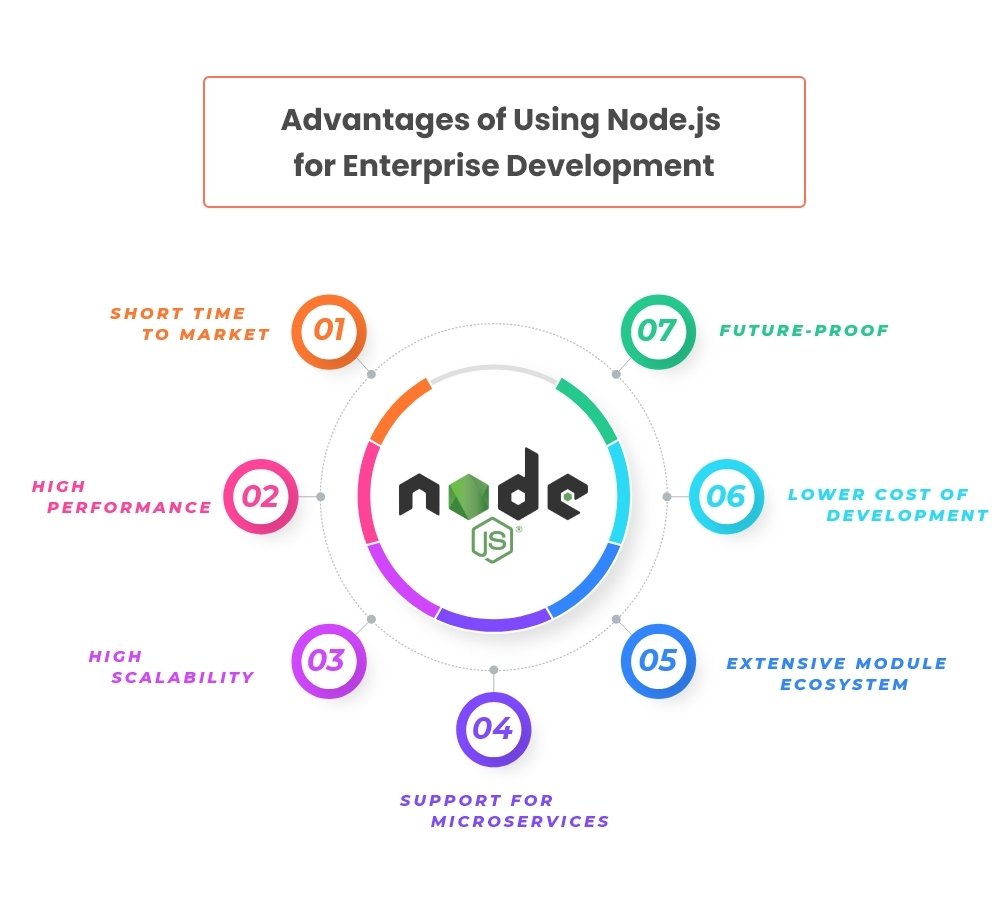
Building a REST API
- Creating a RESTful API with Express
- Define routes for different endpoints (e.g., GET, POST, PUT, DELETE).
- Example: A simple CRUD API for users.
js
Copy code
app.get(‘/users’, (req, res) => {
res.json(users); // Return a list of users
});
- Error Handling and Validation
- Use try-catch blocks for handling errors.
- Validate incoming data using libraries like Joi or express-validator.
Testing and Debugging
- Unit Testing with Mocha and Chai
- Writing tests for your application.
js
Copy code
const chai = require(‘chai’);
const expect = chai.expect;
describe(‘User API’, () => {
it(‘should return all users’, () => {
expect(users).to.be.an(‘array’);
});
});
- Debugging in Node.js
- Using console.log(), debugger, and Node.js built-in debugging tools.
Deploying a Node.js Application
- Deploying on Heroku
- Set up a Heroku account and deploy your Node.js app.
- Set up a Procfile and push the code to Heroku.
- Deploying on DigitalOcean or AWS
Setting up a virtual server and deploying your Node.js app.
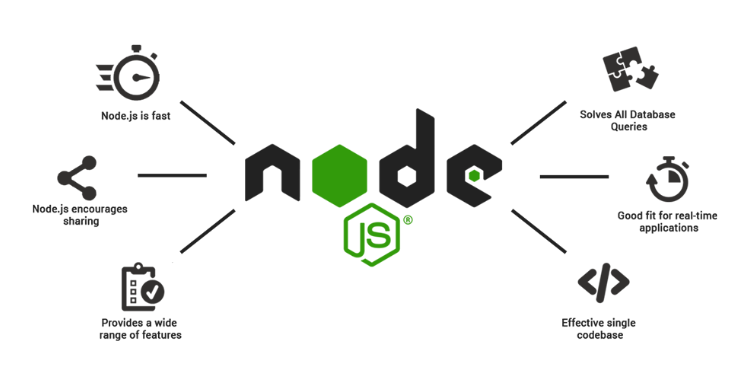
Real-World Application Example
- Building a Simple Chat Application
- Using WebSockets with Socket.io.
- Real-time communication between server and clients.
- Building a To-Do List Application with a Database
- Create a full-stack application with Node.js, Express, and MongoDB.
Additional Topics (Optional)
- GraphQL API with Apollo Server
- Real-time Applications with WebSockets and Socket.io
- Microservices Architecture with Node.js
Conclusion
This Node.js course has covered the fundamentals, including setting up a server, working with databases, building REST APIs, handling asynchronous programming, and deploying applications. With this knowledge, you can now begin building scalable, real-time web applications with Node.js. Continue exploring advanced topics like WebSockets, GraphQL, or microservices to deepen your understanding and skills.
Learning Resources
- Official Node.js Documentation: https://nodejs.org/en/docs/
- Express.js Documentation: https://expressjs.com/
- MongoDB Documentation: https://www.mongodb.com/docs/
Happy coding!