Table of Contents
ToggleReact.js Full Course for Beginners
React.js is a widely used JavaScript library for creating dynamic and interactive user interfaces in modern web applications. If you’re a beginner looking to dive into React, this course will walk you through everything from setting up your environment to understanding core concepts and building real-world applications.
Here’s a structured React.js course outline for beginners that covers the fundamentals of React, including the core concepts, practical examples, and key libraries often used with React.
Module 1: Introduction to React
- Lesson 1.1: What is React?
- Overview of React
- Why React is popular for building modern web apps
- Comparison with other libraries and frameworks (e.g., Angular, Vue)
- Lesson 1.2: Setting Up the Development Environment
- Installing Node.js and npm (Node Package Manager)
- Installing React with Create React App
- Understanding the folder structure in a React project
- Running the development server
Module 2: JSX (JavaScript XML)
- Lesson 2.1: Introduction to JSX
- What is JSX and why it’s used
- JSX syntax basics (tags, attributes, expressions)
- Rendering JSX elements in the DOM
- Lesson 2.2: Using JSX with JavaScript
- Embedding expressions in JSX
- Conditional rendering
- Handling events in JSX (click, submit, etc.)
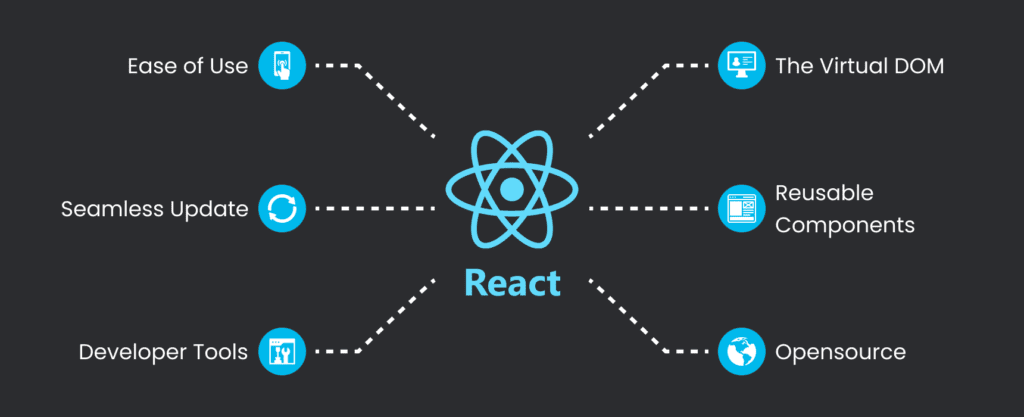
Module 3: React Components
- Lesson 3.1: Understanding Components
- What are components and why they are the building blocks of React applications
- Functional vs Class Components
- Creating and rendering components
- Lesson 3.2: Props (Properties)
- Passing data to components using props
- Understanding prop types
- Default props
- Lesson 3.3: State and Lifecycle
- Introduction to state in React components
- Updating state and handling user interactions
- The useState hook (in functional components)
- Lifecycle methods in class components
- Using the useEffect hook in functional components
Module 4: Handling Events and Forms
- Lesson 4.1: Event Handling in React
- Handling events in React (e.g., click, change, submit)
- Passing arguments to event handlers
- Lesson 4.2: Forms in React
- Creating controlled components for form handling
- Managing form state and input validation
- Submitting forms
Module 5: React Router (for Navigation)
- Lesson 5.1: Introduction to React Router
- What is React Router and why it’s used
- Installing React Router
- Setting up routes and links
- Lesson 5.2: Navigating between Pages
- Defining routes with <Route />
- Using <Link /> and useNavigate for navigation
- Nested routes and dynamic routing
- Lesson 5.3: Route Parameters
- Passing parameters in routes
- Accessing route parameters with useParams
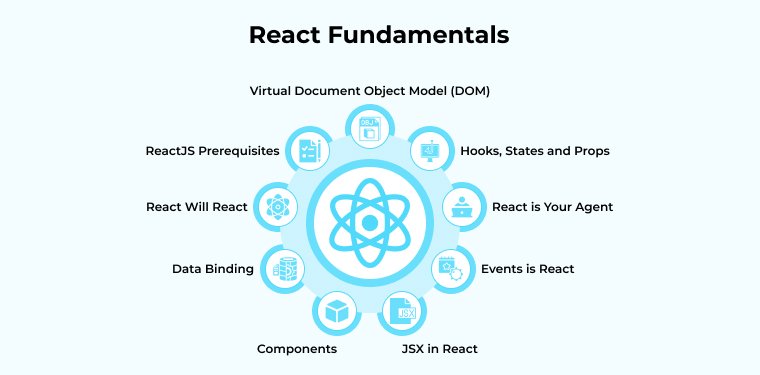
Module 6: Managing State with Hooks
- Lesson 6.1: The useState Hook
- Managing component-level state
- State updates and re-rendering
- Lesson 6.2: The useEffect Hook
- Running side effects (fetching data, setting up subscriptions, etc.)
- Clean-up of side effects
- Lesson 6.3: The useContext Hook (for global state)
- Introduction to Context API
- Passing state across components without prop drilling
Module 7: Component Styling
- Lesson 7.1: Inline Styling in React
- Using JavaScript objects for inline styles
- Handling dynamic styles based on state
- Lesson 7.2: CSS in React
- External CSS files and class names
- Styled Components (CSS-in-JS)
- Lesson 7.3: CSS Modules
- Scoped CSS with CSS modules for better styling management
Module 8: Handling Data (APIs and Fetching Data)
- Lesson 8.1: Fetching Data with fetch()
- Making API requests in React
- Displaying data fetched from an API
- Lesson 8.2: Using axios for API Calls
- Installing and using Axios for HTTP requests
- Error handling with Axios
- Lesson 8.3: Handling Loading States
- Showing loading indicators while fetching data
- Handling errors and retries
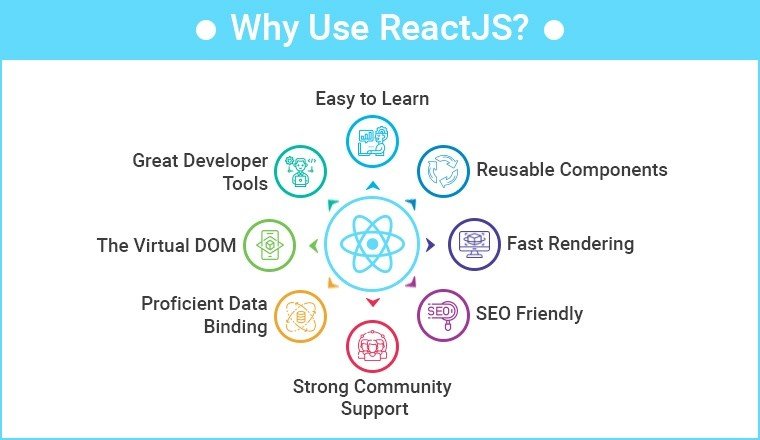
Module 9: Managing Application State (Redux)
- Lesson 9.1: Introduction to Redux
- What is Redux and how it works
- Actions, Reducers, and Store
- Lesson 9.2: Integrating Redux with React
- Setting up Redux in a React application
- Dispatching actions and connecting components
- Lesson 9.3: Using Redux DevTools
- Debugging and inspecting state changes with Redux DevTools
Module 10: Testing React Components
- Lesson 10.1: Introduction to Testing
- Importance of testing React components
- Overview of testing libraries (Jest, React Testing Library)
- Lesson 10.2: Writing Unit Tests for React Components
- Writing tests for functional components and class components
- Mocking events and API calls
Module 11: Advanced Topics
- Lesson 11.1: Code Splitting and Lazy Loading
- Dynamic imports and lazy loading components
- Optimizing performance with React’s Suspense
- Lesson 11.2: Error Boundaries
- Handling errors in React components
- Using Error Boundaries to catch JavaScript errors
- Lesson 11.3: Server-Side Rendering (SSR) and Static Site Generation (SSG)
- Overview of SSR and SSG
- Using Next.js for SSR and SSG with React
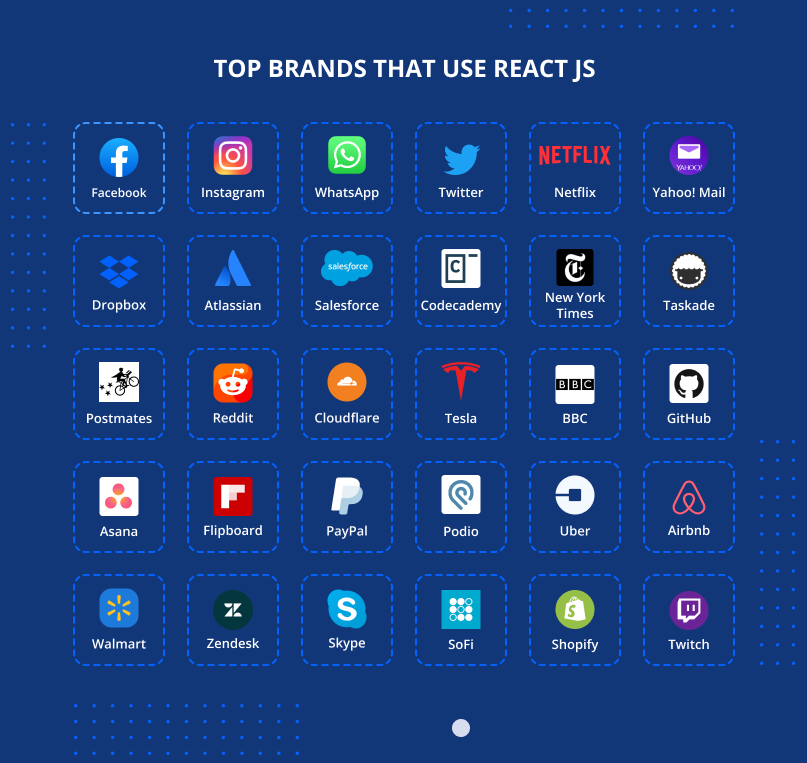
Module 12: Deploying a React Application
- Lesson 12.1: Preparing a React App for Production
- Optimizing React app for deployment
- Building a production-ready app
- Lesson 12.2: Deploying on Platforms (e.g., Netlify, Vercel)
- Step-by-step guide to deploying React apps on Netlify and Vercel
Additional Resources:
- Official Documentation: React Documentation – reactjs.org/docs
- Code Editor: Visual Studio Code – code.visualstudio.com
- React DevTools: React Developer Tools
Tools & Libraries:
- Create React App: To quickly create a new React project.
- React Router: For handling navigation in your app.
- Redux: For state management (optional but highly recommended for larger applications).
- Jest & React Testing Library: For testing your React components.
- Styled Components: For writing CSS in JS.
This course will guide you from understanding the core concepts of React to building and deploying real-world applications.