Table of Contents
ToggleReact JS Interview Questions For Freshers
Here are 30 important React.js interview questions for freshers that can help you prepare for an interview:
What is React.js?
Answer: React.js is a JavaScript library used for building user interfaces, primarily for single-page applications. It enables developers to build reusable UI components and efficiently manage the view layer.
What are the key features of React?
Answer:
- JSX: JavaScript syntax extension for creating React elements.
- Components: Reusable building blocks of a React application.
- Virtual DOM: Efficient way to update the UI by minimizing direct manipulation of the real DOM.
- Unidirectional Data Flow: Data flows in one direction, making state management predictable.
- Hooks: Functions that allow you to use state and lifecycle methods in functional components.
What is JSX in React?
Answer: JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML structures in JavaScript. It makes it easier to visualize and write React components.
What is the difference between props and state in React?
Answer:
- Props: Short for properties, props are used to pass data from a parent component to a child component. Props are immutable.
- State: A state is used to store data that can change over time and can affect how the component renders.
What are functional components in React?
Answer: Functional components are JavaScript functions that return JSX. They are simpler to write and do not have their own state or lifecycle methods (though with hooks, functional components can manage state and lifecycle).
What are class components in React?
Answer Class components are ES6 classes that inherit from React.Component. They can hold and manage state and have lifecycle methods. They are more complex than functional components but have similar functionality.
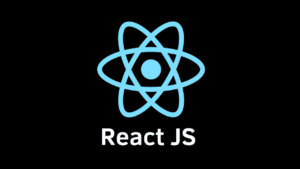
What is the Virtual DOM?
Answer The Virtual DOM is an in-memory representation of the real DOM.React maintains a virtual DOM to improve performance by minimizing direct manipulation of the real DOM. When state or props change, React updates the virtual DOM first, then calculates the difference (reconciliation) and applies only the necessary changes to the real DOM.
What are hooks in React?
Answer: Hooks are functions that allow you to “hook into” React state and lifecycle features from functional components. Some common hooks are:
- useState: is a hook that enables state management in functional components.
- useEffect: For performing side effects in functional components.
- useContext: For consuming context values in functional components.
What is useState hook?
Answer: useState is a hook that enables state management within functional components. It returns an array with two elements: the current state value and a function to update it.
What is useEffect hook?
AnsweruseEffect is a hook that allows you to perform side effects in functional components, like fetching data, subscribing to events, or directly manipulating the DOM. It runs after every render by default.
What is the difference between useEffect and componentDidMount?
Answer: useEffect in functional components can be used for similar purposes as componentDidMount in class components. It runs after every render, but you can control when it runs by passing dependencies as the second argument.
What is JSX compilation in React?
Answer: JSX is not understood by browsers directly. It is compiled into regular JavaScript calls using React’s React.createElement function. This happens during the build process (using tools like Babel).
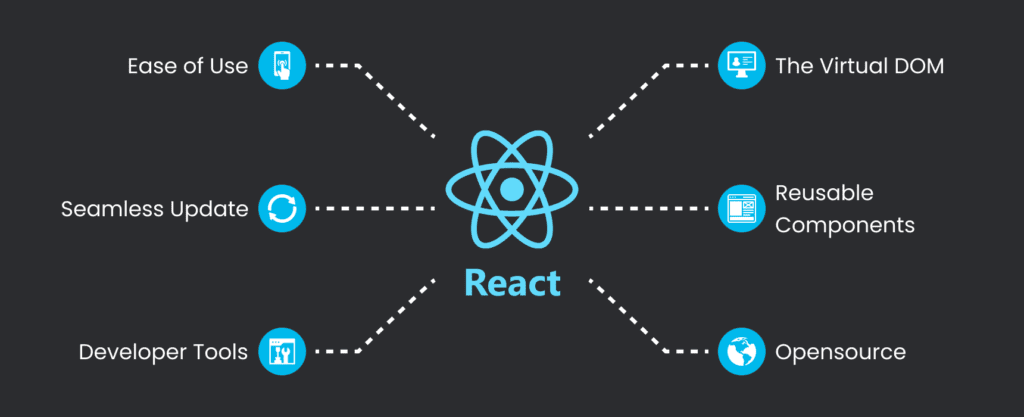
What are React keys and why are they important?
Answer: Keys are unique identifiers used by React to keep track of elements in a list. They help React efficiently update and re-render lists of components by distinguishing between items that have changed.
What is React Router?
Answer: React Router is a library used for routing in React applications. It allows you to navigate between different views or components in a single-page application without reloading the entire page.
What is the difference between componentDidMount() and useEffect()?
Answer: componentDidMount() is a lifecycle method in class components that runs once after the component is mounted. useEffect() serves a similar purpose in functional components and can be configured to run once after mount, on state changes, or on unmount.
What is Context API in React?
Answer: The Context API allows you to share data throughout the component tree without the need to pass props manually at each level. It is used for global state management in a React application.
What are controlled and uncontrolled components?
Answer:
- Controlled Components: A component whose value is controlled by React state (e.g., a form input).
- Uncontrolled Components: A component that maintains its own state internally (e.g., DOM elements like <input> with refs).
What are the advantages of using React?
Answer:
- Reusable components.
- Efficient UI rendering with the Virtual DOM.
- Unidirectional data flow.
- Easy integration with other libraries and frameworks.
- Rich ecosystem and community support.
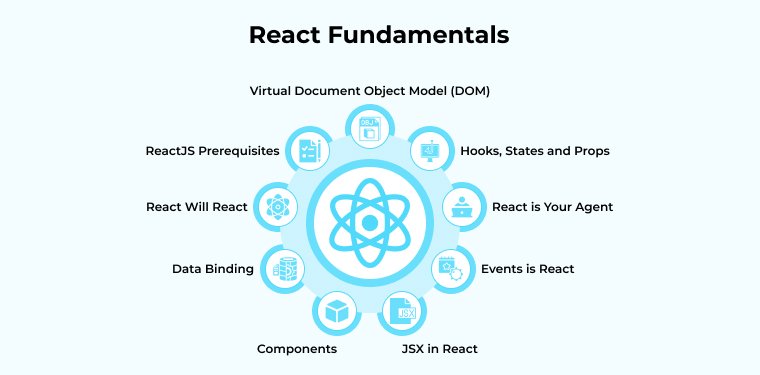
What is the purpose of the render() method in React class components?
Answer: The render() method is required in class components. It returns the JSX that describes the UI for the component.
What is a higher-order component (HOC)?
Answer: A Higher-Order Component is a function that takes a component and returns a new component enhanced with additional props or functionality. It is used for reusing component logic.
How do you optimize performance in React?
Answer: You can optimize performance in React by:
- Using React.memo for functional components.
- Using PureComponent for class components.
- Avoiding unnecessary re-renders by managing state and props efficiently.
- Using code-splitting to load only required code.
What is React’s useReducer hook?
Answer: useReducer is a hook that manages complex state logic in React. It is an alternative to useState when the state depends on multiple actions or needs to be updated in a more controlled manner.
What are React Fragments?
Answer: React Fragments let you group a list of children without introducing additional nodes to the DOM.It’s useful when you want to return multiple elements from a component without a wrapper div.
What is the role of key prop in React?
Answer: The key prop helps React identify which items have changed, are added, or are removed. It is used when rendering lists of components, ensuring efficient re-rendering.
What distinguishes state from props in React?
Answer:
- State is data that a component manages and can change over time.
- Props are read-only data passed from a parent to a child component.
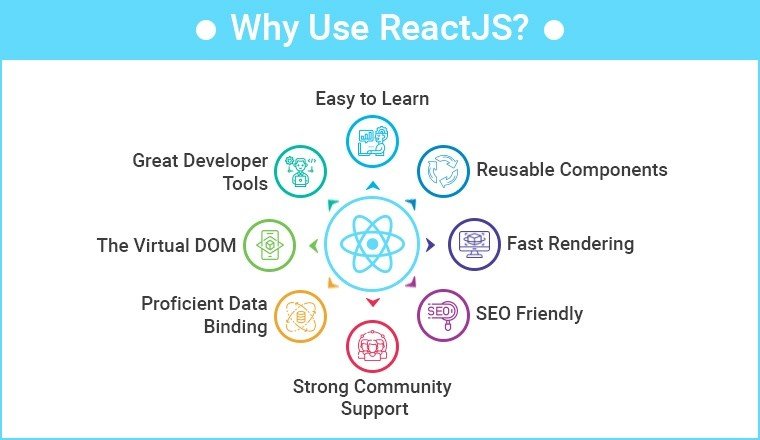
How do you handle events in React?
Answer: You handle events in React by passing event handlers as props to elements (e.g., onClick, onChange). React normalizes events to work across browsers.
What is the purpose of React.StrictMode?
Answer: React.StrictMode is a wrapper component that helps in identifying potential problems in an application, such as deprecated lifecycle methods and other warnings during development.
What is the difference between React.Component and React.PureComponent?
Answer: React.PureComponent is similar to React.Component but implements a shallow comparison of props and state to optimize rendering. It prevents unnecessary re-renders if the props and state have not changed.
What are refs in React?
Answer: Refs are used to access the underlying DOM element or instance of a component. They provide a way to interact directly with DOM elements or class component instances without using state.
What is server-side rendering (SSR) in React?
Answer: Server-side rendering is the process of rendering a React application on the server, sending the fully rendered HTML to the client, and improving the initial load performance and SEO.
These questions and answers should provide a good starting point for React.js interviews for freshers. Practice them well and explore each concept to enhance your understanding of React development.