SELENIUM ADVANCED INTERVIEW QUESTIONS
Here are 30 advanced Selenium interview questions, along with concise explanations, to assist you in preparing for your interview:
- What are the different types of waits in Selenium?
- Implicit Wait: Tells Selenium to wait for a certain amount of time before throwing an exception.
- Explicit Wait: Tells Selenium to wait for a specific condition (like element visibility) to be met before proceeding.
- Fluent Wait: Similar to explicit wait but with the ability to define the polling frequency.
- What is the difference between driver.get() and driver.navigate().to()?
- driver.get(url) is used to open a URL.
- driver.navigate().to(url) is used to navigate to a URL, and it also allows backward and forward navigation, like browser’s back and forward buttons.
- How does Selenium WebDriver interact with JavaScript?
- Selenium WebDriver interacts with JavaScript by executing JavaScript code directly using JavascriptExecutor. The method driver.executeScript() allows you to run JavaScript code in the context of the current browser window.
- What is JavascriptExecutor in Selenium?
- JavascriptExecutor is an interface that allows you to execute JavaScript code in the context of the currently loaded web page. It is commonly used for handling popups, alerts, and dynamic elements.
- What are the different types of locators in Selenium?
- ID: Most reliable and fastest.
- Name: Suitable for elements with a unique name attribute.
- Class Name: Works with the class attribute.
- Tag Name: Used for finding elements by tag name.
- Link Text: Used for anchor (<a>) tags.
- Partial Link Text: Used for matching a substring of link text.
- CSS Selector: Allows using CSS selectors to find elements.
- XPath: A powerful locator strategy based on the XML path.
- What is the difference between XPath and CSS selectors?
- XPath: Can traverse both up and down the DOM tree, allows for more complex queries. Can be slow.
- CSS Selectors: Generally faster than XPath and work only for downward traversal in the DOM.
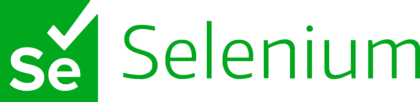
- What is Page Object Model (POM)?
- The Page Object Model (POM) is a design pattern that promotes creating separate classes for each web page in your application. Each class represents a page and has methods for interacting with elements on that page.
- What is Selenium Grid?
- Selenium Grid is a tool that allows you to run tests on multiple machines and browsers in parallel. It helps in distributed testing, speeding up test execution.
- How do you handle browser popups/alerts in Selenium?
- Use the Alert interface in Selenium to interact with browser popups. Methods include accept(), dismiss(), getText(), and sendKeys() for handling alert dialogs.
- What are some ways to handle dynamic elements in Selenium?
- Use dynamic XPath with contains, starts-with, and other attributes.
- Use waits (Explicit or Fluent Waits) to wait for the element to be available.
- What is the difference between driver.quit() and driver.close()?
- driver.quit() closes all windows and ends the WebDriver session.
- driver.close() closes the current window only, without ending the WebDriver session.
- How do you handle drop-downs in Selenium?
- Use the Select class to handle drop-downs. Methods include selectByVisibleText(), selectByIndex(), and selectByValue().
- What is the use of ActionChains in Selenium?
- ActionChains is used to perform advanced user interactions like mouse movements, keyboard actions, drag-and-drop, and right-clicks.
- How can you handle frames in Selenium?
- Use the switchTo() method to switch to a frame. You can switch by index, name, or element.
- Example: driver.switchTo().frame(“frameName”) or driver.switchTo().frame(index).
- What is the use of WebDriverWait in Selenium?
- WebDriverWait is an explicit wait used to wait for a certain condition to be true before proceeding with the next action. It allows you to wait dynamically based on conditions like visibility of elements.
- How can you handle file uploads in Selenium?
- Selenium doesn’t support file uploads through file dialog boxes directly. Use the sendKeys() method to send the file path to file input fields. For more complex scenarios, you may use tools like AutoIT or Robot class.
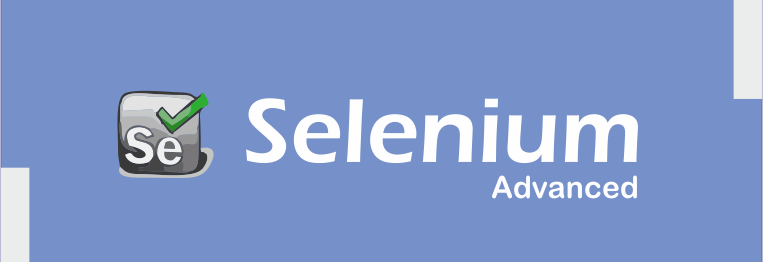
- What is the difference between findElement() and findElements() in Selenium?
- findElement() returns a single WebElement, throws a NoSuchElementException if the element is not found.
- findElements() returns a list of WebElements, and it returns an empty list if no element is found.
- What is a headless browser, and how do you use it in Selenium?
- A headless browser runs without a graphical user interface. It can be used for testing in environments where GUI is not required (like CI/CD). To use a headless browser in Selenium, set browser options like –headless for Chrome or Firefox.
- Explain how to take screenshots in Selenium.
- Selenium WebDriver has the TakesScreenshot interface. You can use it to capture screenshots. Example:
java
Copy code
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
- How do you handle mouse movements and actions in Selenium?
- The Actions class in Selenium is used for simulating mouse movements, keyboard actions, drag-and-drop operations, and other complex user interactions.
- What is the difference between @BeforeTest and @BeforeMethod in TestNG?
- @BeforeTest: Runs before any test methods in the <test> tag in the TestNG XML file.
- @BeforeMethod: Runs before each individual test method.
- What is the use of the Robot class in Selenium?
- The Robot class is used to simulate keyboard and mouse actions at a low level. It’s useful when dealing with OS-level pop-ups and for automating actions outside of the browser.
- How do you handle multiple windows in Selenium?
- Selenium WebDriver provides window handles for switching between multiple browser windows. You can use driver.getWindowHandles() to get all window handles and then switch using driver.switchTo().window(handle).
- Explain the concept of Fluent Wait in Selenium.
- Fluent Wait is an advanced version of Explicit Wait. It allows you to set both the maximum wait time and the frequency with which Selenium should check the condition.
- How would you handle synchronization issues in Selenium?
- Use implicit or explicit waits to handle synchronization problems. Ensure that your tests wait for elements to become visible or interactable before proceeding.
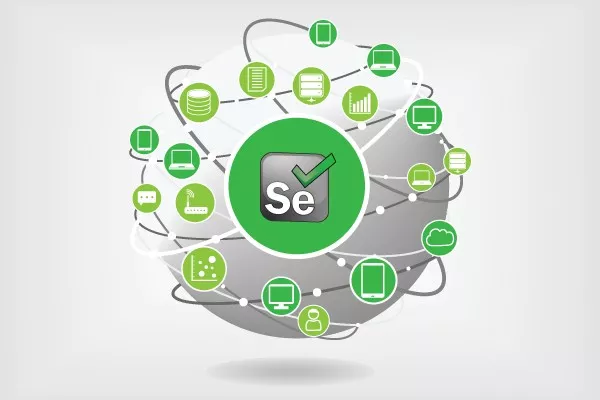
- What is the Page Factory in Selenium?
- Page Factory is an optimization of the Page Object Model (POM) pattern. It uses annotations like @FindBy to locate elements, reducing the need to explicitly use findElement().
- How would you handle a dynamic element that changes its ID every time the page reloads?
- Use XPath with partial matching like contains(@id, ‘someIdPart’) or starts-with(@id, ‘prefix’) to deal with dynamic attributes.
- What is the role of WebDriverListener in Selenium?
- A WebDriverListener is used to capture and log WebDriver events such as test execution lifecycle, capturing browser logs, or reporting errors during the test execution.
- What is the difference between Thread.sleep() and WebDriverWait?
- Thread.sleep() is a static wait that pauses the entire test for a fixed amount of time.
- WebDriverWait is a dynamic wait that waits for a specific condition to be met within a given timeout, making it more efficient.
- How do you perform data-driven testing in Selenium?
- Data-driven testing can be performed using TestNG and tools like Apache POI for reading from Excel, CSV files, or databases. You can use @DataProvider in TestNG to feed different sets of data to your test methods.
These questions cover various aspects of Selenium, from basic to advanced techniques. It’s important to not only understand the theory behind these concepts but also be able to demonstrate them in practical scenarios during the interview.