Table of Contents
ToggleSELENIUM WITH PYTHON
Here’s a detailed look at some important interview questions for Selenium with Python, along with explanations of what interviewers might be looking for in your responses:
What is Selenium, and why is it used?
Selenium is a collection of tools designed for automating web browsers. It’s primarily used for testing web applications to ensure they function as intended. Interviewers want to see your understanding of its purpose and advantages, such as being open-source, supporting multiple programming languages, and enabling cross-browser testing.
Explain the different components of Selenium.
The main components are:
Selenium WebDriver: The core component for browser automation.
Selenium IDE: A tool for recording and playback of tests.
Selenium Grid: enables the parallel execution of tests across multiple machines and browsers, facilitating cross-browser testing in a distributed environment.
Selenium RC (Remote Control): An older component that is largely replaced by WebDriver. Understanding the evolution of these components shows depth in your knowledge.
How do you install Selenium in Python?
You typically install Selenium via pip:
“`bash
pip install selenium
“`
Interviewers may ask this to gauge your familiarity with setting up the environment.
What is WebDriver, and how does it differ from Selenium RC?
WebDriver is an interface for automating web applications, directly communicating with the browser, whereas Selenium RC requires a server to interact with the browser. WebDriver is more efficient and is the preferred approach in modern automation.
Describe different strategies to locate web elements?
Common strategies include:
ID: `driver.find_element(By.ID, “element_id”)`
Name: `driver.find_element(By.NAME, “element_name”)`
XPath: `driver.find_element(By.XPATH, “//tag[@attribute=’value’]”)`
CSS Selector: `driver.find_element(By.CSS_SELECTOR, “tag.class”)`
Understanding these methods shows your ability to work with various types of web elements.
What are implicit and explicit waits? When would you use each?
Implicit Wait: Sets a default wait time for the lifetime of the WebDriver instance, applied to all elements.
Explicit Wait: Allows waiting for specific conditions to be met before proceeding (using `WebDriverWait`).
You should discuss when to use each, like implicit for overall wait time and explicit for dynamic content that may load at different times.
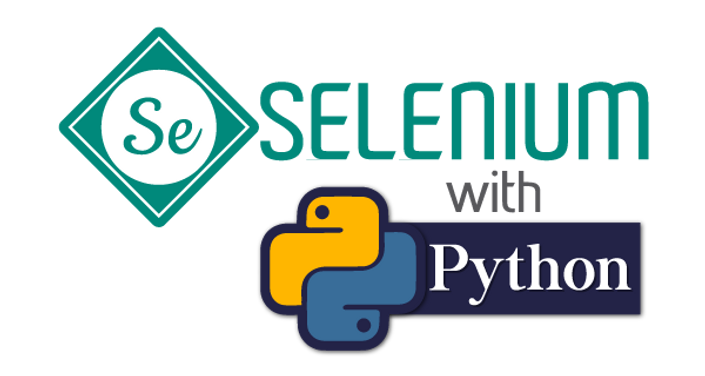
How do you Manage alerts and pop-ups in Selenium?
Use `switch_to.alert` to switch the focus to the alert. Methods like `accept()`, `dismiss()`, and `send_keys()` allow you to interact with alerts. This question checks your knowledge of dealing with unexpected browser behavior.
Explain how to perform actions like mouse hover and drag-and-drop?
Use the `ActionChains` class. For example:
“`python
from selenium.webdriver import ActionChains
action = ActionChains(driver)
action.move_to_element(element).perform() # Mouse hover
action.drag_and_drop(source, target).perform() # Drag and drop
“`
This shows your ability to handle complex user interactions.
How can you take a screenshot in Selenium?
You can use:
“`python
driver.save_screenshot(‘screenshot.png’)
“`
or for more control:
“`python
from PIL import Image
driver.get_screenshot_as_file(‘screenshot.png’)
“`
This tests your knowledge of capturing application state during testing.
What are common exceptions in Selenium, and how do you handle them?
Common exceptions include:
– `NoSuchElementException`
– `TimeoutException`
– `ElementNotInteractableException`
Discussing how to handle these exceptions, perhaps using try-except blocks or implementing retry logic, shows your practical experience.
How do you run tests in parallel using Selenium?
Discuss using Selenium Grid to distribute tests across multiple machines and browsers or leveraging frameworks like pytest with plugins like `pytest-xdist` for parallel execution. This demonstrates your understanding of optimizing test execution time.
Explain the Page Object Model (POM) and its benefits?
POM is a design pattern that separates test logic from the UI structure by creating classes for each page of the application. Benefits include improved maintainability, reusability, and organization. Sharing a brief example can strengthen your answer.
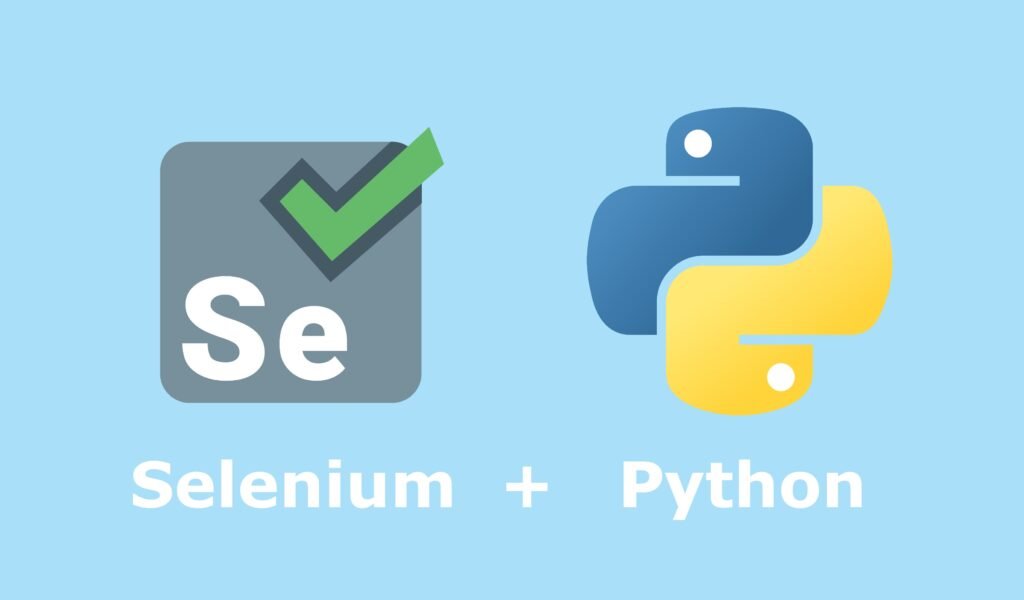
How do you handle dynamic web elements?
Dynamic elements can be handled using explicit waits with conditions like visibility or presence. You might mention using strategies like XPath that accommodate changing attributes. This reflects your ability to adapt tests to real-world scenarios.
Can you write a sample script to automate a simple login process?
Be prepared to write a concise script. This showcases your coding ability and familiarity with Selenium:
“`python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get(‘http://example.com/login’)
driver.find_element(By.NAME, ‘username’).send_keys(‘your_username’)
driver.find_element(By.NAME, ‘password’).send_keys(‘your_password’ + Keys.RETURN)
“`
What strategies do you use for handling flaky tests?
Discuss using explicit waits, ensuring tests run in a clean state, and implementing retries for failed tests. This shows you understand the challenges of test automation and how to address them.
What is WebDriver?
WebDriver is an interface that allows you to write instructions for browsers in a programming language like Python, enabling automation of web applications.
How do you handle waits in Selenium?
There are two types of waits: implicit waits (set a default wait time) and explicit waits (wait for specific conditions). You can use `WebDriverWait` for explicit waits.
How do you locate elements in Selenium?
Elements can be located using various strategies such as `find_element_by_id`, `find_element_by_name`, `find_element_by_xpath`, and more.
What are the differences between `find_element` and `find_elements`?
`find_element` returns a single WebElement, while `find_elements` returns a list of WebElements matching the criteria.
How do you perform actions like mouse hover or drag-and-drop in Selenium?
You can use the `ActionChains` class to perform complex user interactions like mouse hover or drag-and-drop.
How do you take a screenshot in Selenium?
You can use `driver.save_screenshot(‘screenshot.png’)` to take a screenshot of the current page.
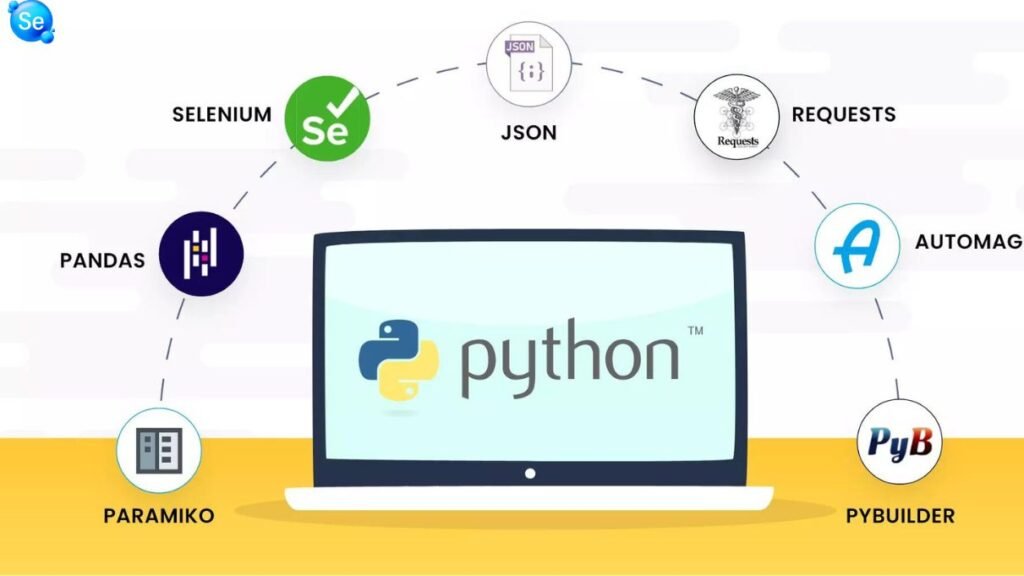
How do you run tests in parallel using Selenium?
You can use Selenium Grid to run tests in parallel on different machines and browsers.
What are the common exceptions you encounter in Selenium?
Common exceptions include `NoSuchElementException`, `TimeoutException`, `ElementNotInteractableException`, etc.
Can you write a simple script to automate a login process?
Interviewers may ask you to demonstrate your coding skills. Be prepared to write a short script that navigates to a login page, fills in credentials, and submits the form.
25. How do you handle dynamic web elements?
Use explicit waits and dynamic locators (like XPath with contains or starts-with) to handle elements that change after the page load.
How would you test a web application with multiple user roles?
You would create test cases for each role and ensure that role-specific functionalities are tested appropriately.
Conclusion
Prepare not just to answer these questions, but also to demonstrate your coding abilities, problem-solving skills, and understanding of best practices in automation testing with Selenium and Python.
Good luck with your interview!