Table of Contents
ToggleWHAT ARE DATA STRUCTURES IN JAVA
Data structures in Java are specialized formats for organizing, managing, and storing data, allowing for efficient access and modification. Understanding these data structures is fundamental for developers, as they provide the means to handle and manipulate data effectively in various applications.
Types of Data Structures in Java
Arrays
Arrays are the simplest form of data structure in Java. They store a fixed-size sequence of elements of the same type in contiguous memory locations. Arrays allow for fast access to their elements via indices, with a time complexity of O(1) for retrieval. However, their fixed size can be a limitation; resizing an array requires creating a new array and copying the elements, which is inefficient.
ArrayList
ArrayList is part of the Java Collections Framework and offers a resizable array implementation. Unlike arrays, ArrayLists can grow dynamically as elements are added. They offer functions for adding, removing, and accessing elements. While accessing elements remains efficient (O(1)), inserting or deleting elements can take O(n) time due to the need to shift elements.
LinkedList
A LinkedList consists of nodes, each containing data and references to the next (and possibly previous) nodes. This structure allows for efficient insertions and deletions at any position (O(1)), but accessing elements by index is slower (O(n)). LinkedLists are beneficial for applications that need frequent modifications to the list.
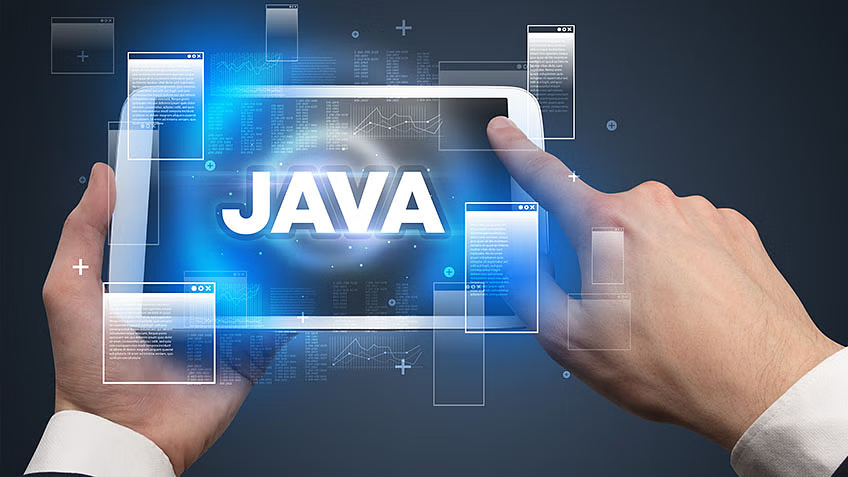
HashMap
HashMap is part of the Java Collections Framework and implements the Map interface. It uses a hash table to store key-value pairs, providing average time complexity of O(1) for retrieval, insertion, and deletion. HashMaps do not maintain the order of elements, which can be a drawback if order is important.
HashSet
HashSet is similar to HashMap but is used to store unique elements without duplicates. It is implemented using a hash table, offering O(1) time complexity for basic operations. However, like HashMap, it does not maintain any order of its elements.
TreeMap
TreeMap is a sorted map implementation that utilizes a red-black tree. It maintains entries in a sorted order based on keys and provides O(log n) time complexity for insertions, deletions, and lookups. This structure is beneficial when you need to keep your data in a specific order.
Stack
A Stack is a last-in, first-out (LIFO) data structure that supports push (adding an element) and pop (removing the top element) operations. Stacks are often employed in algorithms that involve backtracking, including depth-first search.
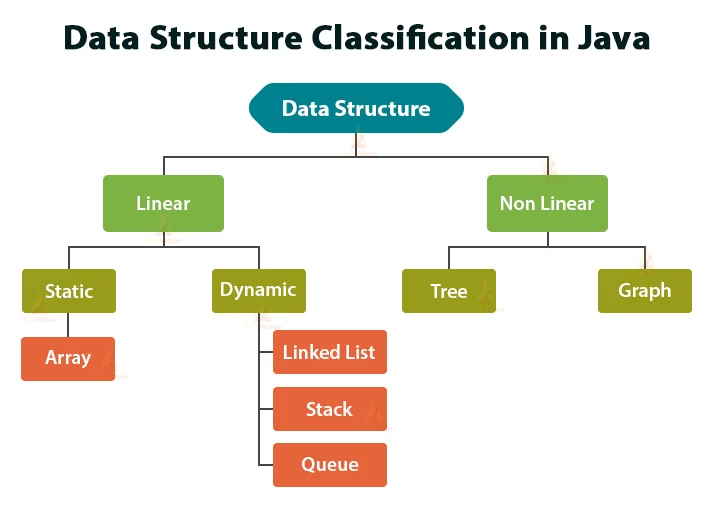
Queue
A Queue is a first-in, first-out (FIFO) data structure. Elements are added at one end (the rear) and removed from the other (the front). Queues are essential in scheduling tasks and breadth-first search algorithms. Java provides various implementations of queues, including LinkedList and PriorityQueue.
PriorityQueue
A PriorityQueue is a type of queue where elements are ordered based on their priority. The element with the highest priority is removed first, making it useful in scenarios like task scheduling and implementing Dijkstra’s algorithm.
Deque
Deque (double-ended queue) allows insertion and removal of elements from both ends. It can function as both a stack and a queue, offering great flexibility for various applications.
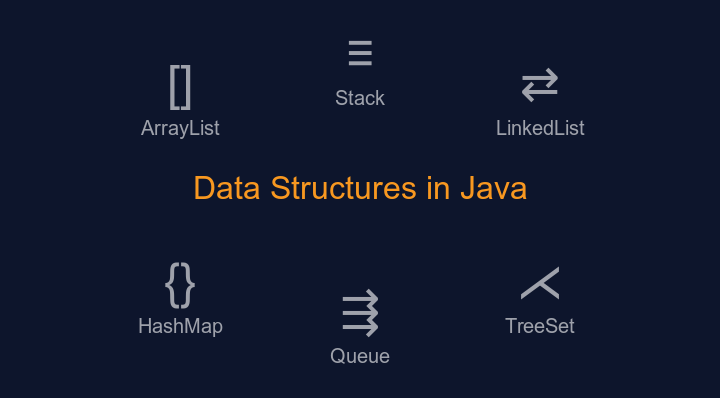
Conclusion
Choosing the right data structure in Java is crucial for optimizing performance and resource utilization. Each data structure has its strengths and weaknesses, making it essential to align the data structure with the specific requirements of your application. By understanding and leveraging these data structures, developers can create efficient and effective programs that handle data appropriately.