Table of Contents
Togglethe OOPs Concept In Selenium
In Selenium, Object-Oriented Programming (OOP) concepts are highly valuable for designing test automation frameworks. By incorporating OOP principles, Selenium scripts can become more modular, maintainable, and scalable. Below, we explore the key OOP concepts applied in Selenium, including Class, Object, Inheritance, Encapsulation, Polymorphism, and Abstraction, and how they contribute to building robust Selenium test scripts.
In object-oriented programming, a class is a blueprint for creating objects (instances). A class defines the properties (variables) and methods (functions) that the objects created from it will possess. In Selenium, a class can represent a particular page of an application or a test case, encapsulating the actions and elements related to that page or test.
For example, in Selenium, you might have a class for the Login page, which contains methods for interacting with the elements on that page (e.g., entering the username and password). By organizing related actions into classes, you make the code modular and easier to maintain.
Example of a Class in Selenium:
java
Copy code
public class LoginPage {
private WebDriver driver;
private WebElement usernameField;
private WebElement passwordField;
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
this.usernameField = driver.findElement(By.id(“username”));
this.passwordField = driver.findElement(By.id(“password”));
this.loginButton = driver.findElement(By.id(“login”));
}
public void enterUsername(String username) {
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
passwordField.sendKeys(password);
}
public void clickLoginButton() {
loginButton.click();
}
}
In this example, LoginPage is a class that defines the web elements and actions specific to the login page, encapsulating the details of interacting with the page.
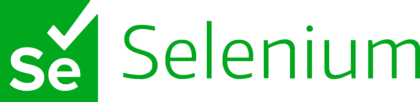
An object is an instance of a class. Objects are created based on the class structure and can access the attributes and methods defined in that class. In Selenium, objects are used to interact with the web elements defined in the class. When a class is instantiated, an object allows you to perform actions like filling out a form or clicking a button.
Example of Creating an Object in Selenium:
java
Copy code
public class LoginTest {
private WebDriver driver;
@Before
public void setUp() {
driver = new ChromeDriver();
driver.get(“https://example.com/login”);
}
@Test
public void testLogin() {
LoginPage loginPage = new LoginPage(driver); // Creating an object of LoginPage
loginPage.enterUsername(“admin”);
loginPage.enterPassword(“password”);
loginPage.clickLoginButton();
}
@After
public void tearDown() {
driver.quit();
}
}
Here, LoginPage loginPage = new LoginPage(driver) creates an object of the LoginPage class, allowing interaction with the login page elements.
Inheritance is a mechanism where a class (child class) inherits properties and behaviors (methods) from another class (parent class). This helps in code reusability, as common functionality can be placed in the parent class, and specific behavior can be implemented in the child class.
In Selenium, inheritance is useful for creating a base class that holds common setup and teardown logic (such as WebDriver initialization) and then having test-specific classes inherit from this base class.
Example of Inheritance in Selenium:
java
Copy code
public class BaseTest {
protected WebDriver driver;
@Before
public void setUp() {
driver = new ChromeDriver();
driver.get(“https://example.com”);
}
@After
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
public class LoginTest extends BaseTest {
@Test
public void testLogin() {
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername(“admin”);
loginPage.enterPassword(“password”);
loginPage.clickLoginButton();
}
}
In this case, the LoginTest class inherits from BaseTest to reuse the WebDriver setup and teardown methods, avoiding repetition.
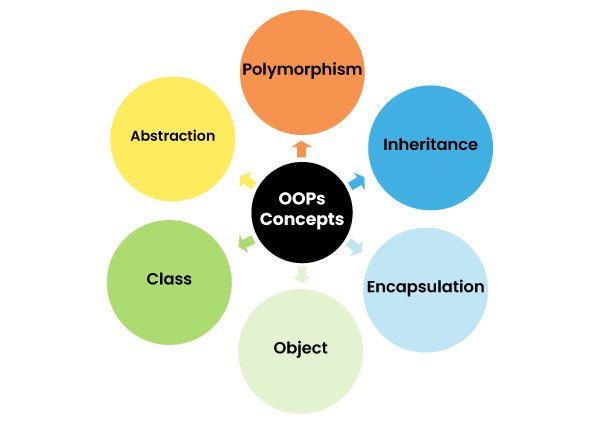
Encapsulation is the practice of bundling data (variables) and the methods that operate on that data into a single unit or class. It also involves restricting access to some of an object’s components, typically by making fields private and providing public getter and setter methods. This allows controlled access to the object’s state.
In Selenium, encapsulation is important for hiding the details of how interaction with web elements occurs, providing a clean interface for interacting with those elements. For instance, you might make WebDriver elements private within a page class and expose methods that interact with those elements.
Example of Encapsulation in Selenium:
java
Copy code
public class LoginPage {
private WebDriver driver;
private WebElement usernameField;
private WebElement passwordField;
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
usernameField = driver.findElement(By.id(“username”));
passwordField = driver.findElement(By.id(“password”));
loginButton = driver.findElement(By.id(“login”));
}
public void enterUsername(String username) {
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
passwordField.sendKeys(password);
}
public void clickLoginButton() {
loginButton.click();
}
}
Here, the WebDriver elements usernameField, passwordField, and loginButton are private, and access to them is provided through public methods like enterUsername() and clickLoginButton(). This encapsulates the details of how Selenium interacts with these elements.
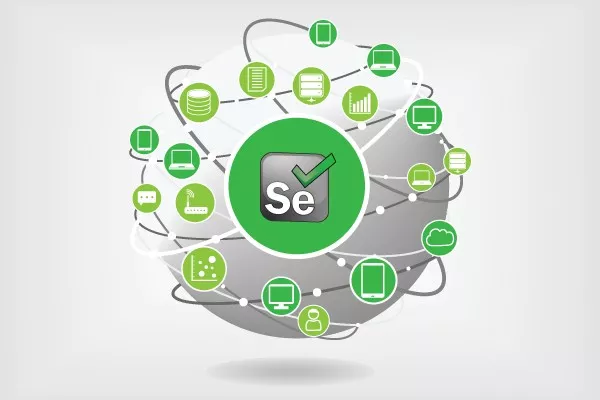
Polymorphism means “many forms” and allows a single method or function to behave differently based on the object that invokes it. It allows for method overriding and method overloading.
In Selenium, polymorphism can be applied in different ways, including overriding methods in child classes or using different strategies for waiting, element interactions, etc. Polymorphism allows for flexibility in writing reusable and dynamic code that adapts to various scenarios.
Example of Polymorphism in Selenium:
java
Copy code
public class WebDriverUtility {
public void waitForElement(WebDriver driver, By locator) {
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(locator));
}
}
public class FluentWaitUtility extends WebDriverUtility {
@Override
public void waitForElement(WebDriver driver, By locator) {
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
wait.until(ExpectedConditions.visibilityOfElementLocated(locator));
}
}
In this case, the method waitForElement is overridden in the subclass FluentWaitUtility to provide a different implementation of the wait mechanism.
Abstraction is the process of hiding the complexity and exposing only the essential features of an object. In Selenium, abstraction can be achieved using abstract classes and interfaces, which define the essential behaviors without specifying the concrete implementation.
For example, you can create a Page interface with methods like loadPage() and verifyPage(), and then concrete page classes like LoginPage can implement these methods to interact with the specific page.
Example of Abstraction in Selenium:
java
Copy code
public interface Page {
void loadPage();
void verifyPage();
}
public class LoginPage implements Page {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
@Override
public void loadPage() {
driver.get(“https://example.com/login”);
}
@Override
public void verifyPage() {
Assert.assertTrue(driver.findElement(By.id(“username”)).isDisplayed());
}
}
In this example, the Page interface abstracts the common page behavior, while LoginPage provides the specific implementation.
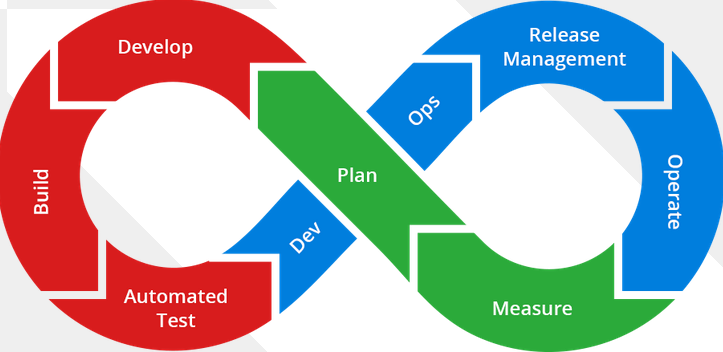
Conclusion
In Selenium, the application of Object-Oriented Programming (OOP) concepts like Class, Object, Inheritance, Encapsulation, Polymorphism, and Abstraction enables testers and developers to create modular, reusable, and maintainable automation code. These OOP principles foster cleaner test designs and improve the scalability and readability of Selenium-based frameworks. By adopting OOP, teams can build flexible automation scripts that are easier to modify and extend as the application evolves.