The `zip` function in Python is a built-in function used to combine multiple iterables (like lists or tuples) into a single iterable of tuples. Each tuple contains elements from the input iterables that are paired together based on their position.
Table of Contents
ToggleKey Features of `zip`
- Combines Iterables: It can take two or more iterables and combines them element-wise.
- Returns an Iterator: The result is an iterator of tuples, which can be converted to a list or used in a loop.
- Stops at the Shortest Iterable: If the input iterables are of different lengths, `zip` stops creating tuples when the shortest iterable is exhausted.
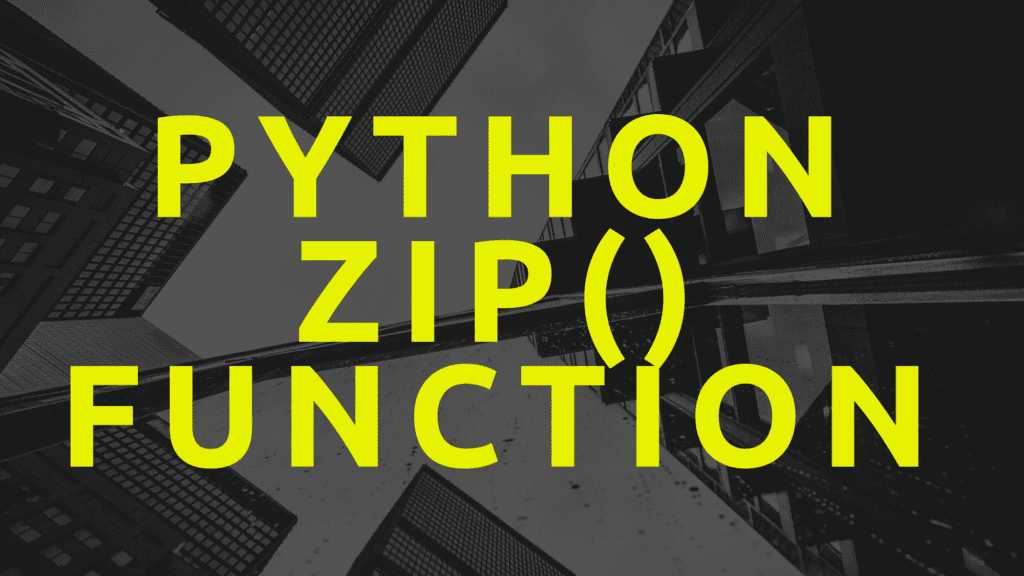
Basic Syntax
“`python
zip(*iterables)
“`
Examples
- Combining Lists
“`python
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’, ‘c’]
zipped = zip(list1, list2)
print(list(zipped)) # Output: [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
- Handling Uneven Lengths
“`python
list3 = [1, 2]
list4 = [‘a’, ‘b’, ‘c’]
zipped_uneven = zip(list3, list4)
print(list(zipped_uneven)) # Output: [(1, ‘a’), (2, ‘b’)]
“`
- Unzipping Values
You can unzip a list of tuples back into separate lists:
“`python
zipped_list = [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
unzipped = zip(*zipped_list)
list1, list2 = map(list, unzipped)
print(list1) # Output: [1, 2, 3]
print(list2) # Output: [‘a’, ‘b’, ‘c’]
“`
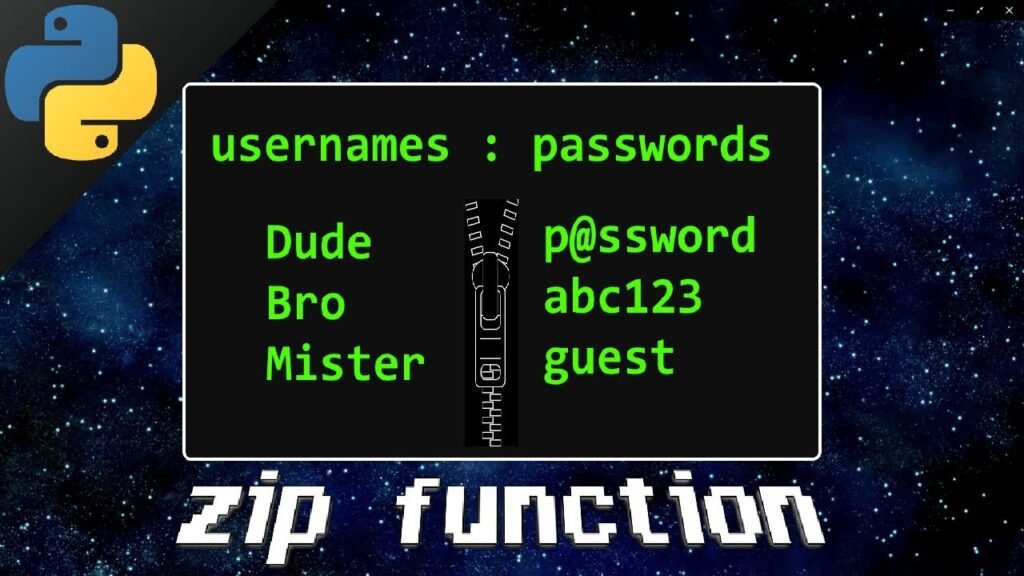
- Common Use Cases
– Creating dictionaries from two lists:
“`python
keys = [‘name’, ‘age’, ‘city’]
values = [‘Alice’, 30, ‘New York’]
dictionary = dict(zip(keys, values))
print(dictionary) # Output: {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
“`
– Iterating over multiple sequences in parallel:
“`python
for num, letter in zip(list1, list2):
print(num, letter)
“`
- Creating Dictionaries
You can use `zip` to create a dictionary from two lists:
“`python
keys = [‘name’, ‘age’, ‘city’]
values = [‘Alice’, 30, ‘New York’]
dictionary = dict(zip(keys, values))
print(dictionary) # Output: {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
“`
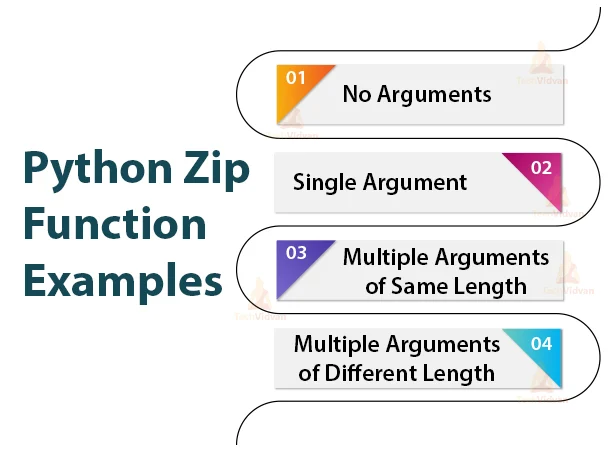
- Iterating in Parallel
You can iterate over multiple sequences at the same time:
“`python
for num, letter in zip(list1, list2):
print(num, letter)
“`
How It Works
– The `zip` function takes multiple iterables as arguments and returns an iterator of tuples.
– The first tuple contains the first elements from each iterable, the second tuple contains the second elements, and so on.
– If the input iterables are of uneven length, `zip` stops creating tuples when the shortest input iterable is exhausted.
Example Usage
“`python
# Example with two lists
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’, ‘c’]
zipped = zip(list1, list2)
print(list(zipped)) # Output: [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
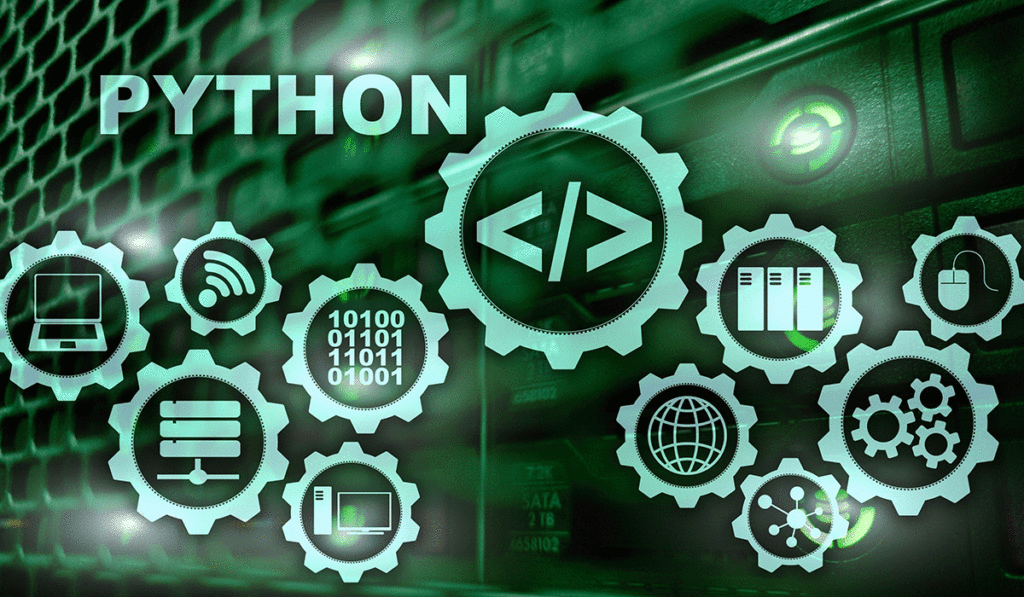
Working with Uneven Lengths
“`python
# Example with uneven lengths
list3 = [1, 2]
list4 = [‘a’, ‘b’, ‘c’]
zipped_uneven = zip(list3, list4)
print(list(zipped_uneven)) # Output: [(1, ‘a’), (2, ‘b’)]
“`
Unzipping
You can also unzip a list of tuples back into separate lists using the `zip` function with the unpacking operator `*`:
“`python
zipped_list = [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
unzipped = zip(*zipped_list)
list1, list2 = map(list, unzipped)
print(list1) # Output: [1, 2, 3]
print(list2) # Output: [‘a’, ‘b’, ‘c’]
“`
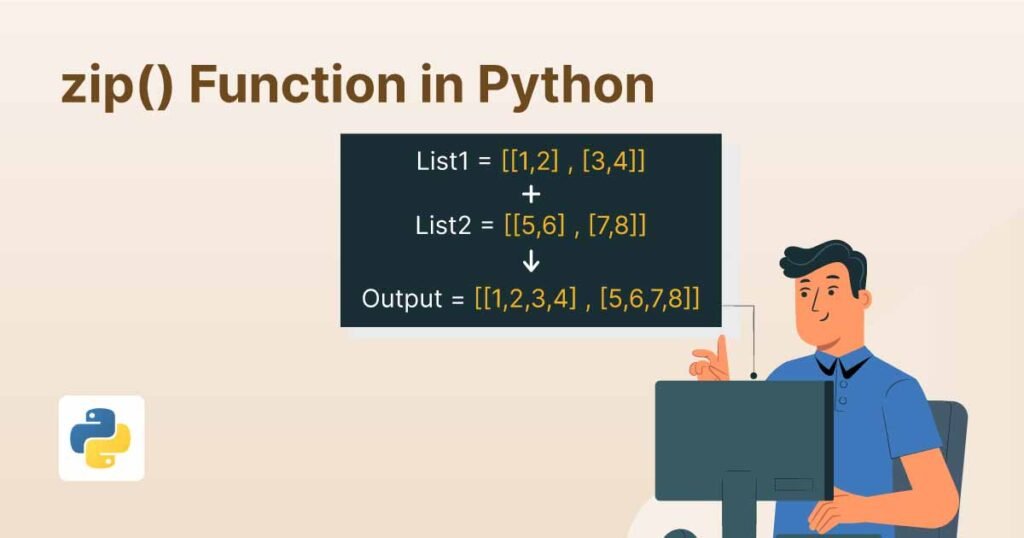
Common Use Cases
– Combining data from multiple sources (like parallel lists).
– Creating dictionaries from two lists (keys and values).
– Iterating over multiple sequences in parallel.
The `zip` function is a handy tool for data manipulation in Python!
Summary
The `zip` function is a powerful tool for combining and manipulating iterables in Python, making it easier to work with related data efficiently.